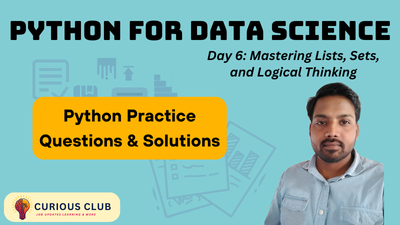
Welcome back to Day 6 of Learning Python for Data Science journey! In the last article, we explored:
✅ Lists
✅ Tuples
✅ Sets
✅ Dictionaries
Now, it’s time to solve the practice questions given in the previous article.
Each question is followed by a detailed explanation and output.
Create a list of five student names and print it.
student = ['Jacob', 'Jenny', 'John', 'Julie']
print(student)
Output
['Jacob', 'Jenny', 'John', 'Julie']
Add a new student name to the end of the list using the appropriate method.
student.append('Mark')
print(student)
Output
['Jacob', 'Jenny', 'John', 'Julie', 'Mark']
Insert a student name at the second position in the list.
student.insert(1, "David")
print(student)
Output
['Jacob', 'David', 'Jenny', 'John', 'Julie', 'Mark']
Remove a specific student name from the list.
student.remove('David')
print(student)
Output
['Jacob', 'Jenny', 'John', 'Julie', 'Mark']
Remove and return the last element of the list.
removed = student.pop()
print('Removed: ', removed)
Output
Removed: Mark
Retrieve a sublist containing elements from index 2 to 4 using slicing.
sublist = student[2:5]
print(sublist)
Output
['John', 'Julie']
Find the index of a specific student name in the list.
index = student.index('Julie')
print(index)
Output
3
Sort a list of integers in ascending order.
numbers = [5, 2, 9, 1, 5, 6]
numbers.sort()
print(numbers)
Output
[1, 2, 5, 5, 6, 9]
Reverse the order of elements in a list.
numbers.reverse()
print(numbers)
Output
[9, 6, 5, 5, 2, 1]
Count the number of times a specific element appears in a list.
count = numbers.count(5)
print('5 appears ',count,' times in the list' )
Output
5 appears 2 times in the list
Find the length of a given list.
length = len(numbers)
print(length)
Output
6
Create a set containing five unique numbers and print it.
num = {23, 45, 67, 89, 78}
print(num)
Output
{67, 23, 89, 45, 78}
Add a new element to the set.
num.add(34)
print(num)
Output
{34, 67, 23, 89, 45, 78}
Try adding a duplicate element to the set and observe the output.
num.add(34)
print(num)
Output
{34, 67, 23, 89, 45, 78}
Set does not store duplicates and removes them.
Remove an element from the set using the appropriate method.
num.remove(34)
print(num) # removed 34
Output
{67, 23, 89, 45, 78}
Perform a union operation between two sets and print the result.
num1 = {12, 23, 34, 12, 34, 45, 67}
num2 = {12, 23, 78, 89, 67, 56, 22}
union = num1.union(num2)
print(union)
Output
{34, 67, 12, 45, 78, 22, 23, 56, 89}
Find the common elements between two sets using the intersection method.
common = num1.intersection(num2)
print(common)
Output
{67, 12, 23}
Find the elements that exist in the first set but not in the second set using the difference method.
difference = num1.difference(num2)
print(difference)
Output
{34, 45}
Check if one set is a subset of another set.
is_subset = num1.issubset(num2)
print(is_subset)
Output
False
Check if two sets have no common elements using the appropriate method.
print('num1: ',num1)
print('num2: ',num2)
print(num1.isdisjoint(num2))
Output
num1: {34, 67, 23, 12, 45}
num2: {67, 22, 23, 56, 89, 12, 78}
False
Write a Python program to count the number of occurrences of each element in a given list.
el = ['R', 'R', 'R', 'S', 'S', 'Sh', 'Sh']
count = {}
for i in el:
if i in count:
count[i] += 1
else:
count[i] = 1
print(count)
Output
{'R': 3, 'S': 2, 'Sh': 2}
Create a set of numbers from 1 to 10. Find all the numbers that are divisible by 3 using a set operation.
nums = {i for i in range(1,11) if i%3 == 0}
print(nums)
Output
{9, 3, 6}
Given two lists of integers, write a program to find the elements that are common in both lists using a set.
set1 = [1, 2, 3, 4, 5, 6]
set2 = [3, 4, 5, 6, 7, 8]
set(set1).intersection(set2)
Output
{3, 4, 5, 6}
Write a program to remove duplicate words from a given string and display the unique words in alphabetical order.
string = ' the boy is quite a boy'
string = set(string.split())
unique = []
result = [i for i in string if i not in unique]
print(result)
Output
['the', 'a', 'is', 'quite', 'boy']
Create a list of mixed data types (e.g., numbers and strings). Write a program to separate the strings and numbers into two different lists.
l = [1, 2, 3, 4, 5, 6, 'R', 'R', 'R', 'S', 'S', 'Sh', 'Sh']
numbers = []
strings= []
for i in l:
if isinstance(i, str):
strings.append(i)
else:
numbers.append(i)
print(numbers, strings)
Write a function that returns the second largest number from a list of integers. Example: [10, 20, 4, 45, 99]
→ Output: 45
num = [10, 20, 4, 45, 99]
num.sort(reverse=True)
print(num)
print('Second largest number is: ',num[1])
Output
[99, 45, 20, 10, 4]
Second largest number is: 45
Given a list and an integer k
, shift elements to the right by k
positions. Example: ([1, 2, 3, 4, 5], k=2)
→ Output: [4, 5, 1, 2, 3]
list1 = [1, 2, 3, 4, 5]
k = 2
new_list = list1[k:] + list1[:k]
print(new_list)
Output
[3, 4, 5, 1, 2]
Given a list, remove duplicate elements while maintaining the order. Example: [1, 2, 2, 3, 4, 4, 5]
→ Output: [1, 2, 3, 4, 5]
l = [1, 2, 2, 3, 4, 4, 5]
l1 = []
[l1.append(i) for i in l if i not in l1]
print(l1)
Output
[1, 2, 3, 4, 5]
Given a list and a target sum, find all unique pairs whose sum equals the target. Example: ([1, 2, 3, 4, 5, 6], target=7)
→ Output: [(1, 6), (2, 5), (3, 4)]
# method 1
lis = [1, 2, 3, 4, 5, 6]
target = 7
result = []
for i in lis:
for j in lis:
if i + j == target and (j, i) not in result:
result.append((i, j))
print(result)
# method 2
re = []
[re.append((i, j)) for i in lis for j in lis if i + j == target and (j, i) not in re]
re
Output
[(1, 6), (2, 5), (3, 4)]
Implement a function that finds the index of a given element in a list manually. Example: ([10, 20, 30, 40], target=30)
→ Output: 2
# defining the function
def position(lis, target):
for i, el in enumerate(lis):
if el == target:
return i
# test data
lis = [10, 20, 30, 40]
target = 30
# calling the function
position(lis, target)
Output
2
Write a function that returns elements that exist in either of the two sets but not both. Example: {1, 2, 3, 4}
and {3, 4, 5, 6}
→ Output: {1, 2, 5, 6}
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
set1.symmetric_difference(set2)
Output
{1, 2, 5, 6}
Given two sets, determine if one set is a subset of the other. Example: {1, 2}
is a subset of {1, 2, 3, 4}
→ Output: True
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
set1.issubset(set2)
Output
False
set1 = {1,2}
set2 = {1,2,3,4,5}
set1.issubset(set2)
Output
True
Given multiple sets, find the total count of unique elements across all sets. Example: [{1, 2, 3}, {3, 4, 5}, {5, 6, 7}]
→ Output: 7
sets = [{1, 2, 3}, {3, 4, 5}, {5, 6, 7}]
set1 = sets[0].union(sets[1]).union(sets[2])
print('total count of unique elements across all sets is : ',len(set1))
Output
total count of unique elements across all sets is: 7
Given three sets, find the elements that are common in all three. Example: {1, 2, 3}
, {2, 3, 4}
, {3, 4, 5}
→ Output: {3}
set1 = {1, 2, 3}
set2 = {2, 3, 4}
set3 = {3, 4, 5}
common = set1.intersection(set2).intersection(set3)
print('element that is common in all three: ',common)
Output
element that is common in all three: {3}
Remove all elements of set2
from set1
without using a loop. Example: {1, 2, 3, 4, 5}
minus {3, 4}
→ Output: {1, 2, 5}
set1 = {1, 2, 3, 4, 5}
set2 = {3, 4}
set1.difference(set2) #1
set1-set2 #2
Output
{1, 2, 5}
Given a list of integers, find the length of the longest consecutive elements sequence. Example: [100, 4, 200, 1, 3, 2] → Output: 4 ([1, 2, 3, 4] is the longest sequence)¶
lis = [100, 4, 200, 1, 3, 2]
re = []
final = []
for i in lis:
if i-1 in lis or i == min(lis):
re.append(i)
re
Output
[4, 1, 3, 2]
Rearrange a sorted list so that the first element is the smallest, the second is the largest, the third is the second smallest, and so on. Example: [1, 2, 3, 4, 5, 6, 7] → Output: [1, 7, 2, 6, 3, 5, 4]
lis = [1, 2, 3, 4, 5, 6, 7]
output = []
while len(lis)>1:
min_val = min(lis)
max_val = max(lis)
output.append(min_val)
output.append(max_val)
lis.remove(min_val)
lis.remove(max_val)
if lis:
output.append(lis[0])
output
Output
[1, 7, 2, 6, 3, 5, 4]
An element is a majority if it appears more than n/2 times in a list of size n. Find it in O(n) time. Example: [3, 3, 4, 2, 4, 4, 2, 4, 4] → Output: 4
def majority_element(nums):
candidate, count = None, 0
# Step 1: Find candidate
for num in nums:
if count == 0:
candidate = num
count += (1 if num == candidate else -1)
# Step 2: Verify candidate
if nums.count(candidate) > len(nums) // 2:
return candidate
return None # No majority element found
# Example usage
lis = [3, 3, 4, 2, 4, 4, 2, 4, 4]
print(majority_element(lis))
Output
4
Given two sets of numbers, find all pairs (a, b) where a is from set1, b is from set2, and (a + b) is even. Example: {1, 2, 3} and {4, 5, 6} → Output: {(2, 4), (2, 6), (1, 5), (3, 5)}
set1 = {1, 2, 3}
set2 = {4, 5, 6}
set3 = set()
for a in set1:
for b in set2:
if (a + b) % 2 == 0:
set3.add((a, b))
print(set3)
Output
{(2, 4), (2, 6), (3, 5), (1, 5)}
We hope this article was helpful for you and you learned a lot about data analyst interview from it. If you have friends or family members who would find it helpful, please share it to them or on social media.
Join our social media for more.
Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your Understanding
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.