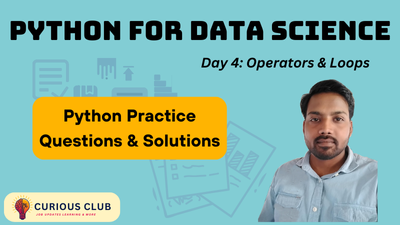
Welcome back to Day 4 of Learning Python for Data Science! In the last article, we explored:
✅ Comparison Operators
✅ Logical Operators
✅ Loops in Python
✅ Loop Control Statements
Now, it’s time to solve the practice questions given in the previous article.
Each question is followed by a detailed explanation and output.
What will be the output of this code?
python
print(10 == 10)
Solution:
This checks if 10
is equal to 10
, which is True
.
Output:
True
Write a Python program that checks whether a number is positive, negative, or zero.
Solution:
num = int(input("Enter a number: "))
if num > 0:
print("Positive Number")
elif num < 0:
print("Negative Number")
else:
print("Zero")
Example Input/Output:
Enter a number: 5
Positive Number
What will be the output?
print(5 > 3 and 10 > 5)
Solution:
Both conditions 5 > 3
and 10 > 5
are True
, so the result is True
.
Output:
True
Write a program to check if a number is divisible by both 2 and 5 using logical operators.
Solution:
num = int(input("Enter a number: "))
if num % 2 == 0 and num % 5 == 0:
print("Divisible by both 2 and 5")
else:
print("Not divisible by both")
Example Input/Output:
Enter a number: 10
Divisible by both 2 and 5
What will be the output of this while
loop?
x = 1
while x < 5:
print(x)
x += 1
Solution:
The loop runs while x
is less than 5, printing 1, 2, 3, 4
.
Output:
1
2
3
4
Modify the code below to use a for
loop instead of while
:
Solution:
for x in range(1, 6):
print(x)
Output:
1
2
3
4
5
What happens if the break
statement is removed from the following loop?
for i in range(1, 6):
if i == 4:
break
print(i)
Solution:
Without break
, the loop prints all numbers from 1 to 5 instead of stopping at 4
.
Output without break
:
1
2
3
4
5
Write a program that prints all even numbers from 1 to 20 using a loop.
Solution:
for i in range(1, 21):
if i % 2 == 0:
print(i, end=" ")
Output:
2 4 6 8 10 12 14 16 18 20
What will be the output of the following?
for i in range(1, 6):
if i == 3:
continue
print(i)
Solution:
The continue
statement skips printing 3
.
Output:
1
2
4
5
Write a Python program that skips all numbers divisible by 3 in a loop from 1 to 10 using continue
.
Solution:
for i in range(1, 11):
if i % 3 == 0:
continue
print(i, end=" ")
Output:
1 2 4 5 7 8 10
Write a for
loop that prints numbers from 1 to 10 but stops when it reaches 7.
Solution:
for i in range(1, 11):
if i == 7:
break
print(i)
Output:
1
2
3
4
5
6
What will be the output?
x = 5
y = 10
print(x > 2 and y < 15)
Solution:
Both conditions are True
, so the result is True
.
Output:
True
Write a program that calculates the sum of numbers from 1 to 100 using a for
loop.
Solution:
total = 0
for i in range(1, 101):
total += i
print("Sum:", total)
Output:
Sum: 5050
Modify the following loop to stop execution when x
is greater than 50.
Solution:
x = 1
while x < 100:
if x > 50:
break
print(x, end=" ")
x += 5
Output:
1 6 11 16 21 26 31 36 41 46
Write a program that counts how many even numbers exist between 1 and 50.
Solution:
count = 0
for i in range(1, 51):
if i % 2 == 0:
count += 1
print("Total even numbers:", count)
Output:
Total even numbers: 25
Modify the following loop to use pass
when x == 5
.
Solution:
for x in range(10):
if x == 5:
pass # Placeholder
else:
print(x)
Output:
0
1
2
3
4
6
7
8
9
Print even numbers from 1 to 20 using a for loop.
for i in range(1,21):
if i % 2 == 0:
print(i)
else:
continue
Output
2
4
6
8
10
12
14
16
18
20
Print the sum of numbers from 1 to 50 using a while loop.
num = 1
sum = 0
while num <=50:
sum += num
num += 1
print(sum)
Output
1275
Print the reverse of a given string using a loop.
name = 'Vishal'
rev = ''
for i in name:
rev = i + rev
print(rev)
Output
lahsiV
Explanation In this code when loop is accesing the first charecter from name it gets 'V'
and stores in rev
. in the next iteration it get i
and since condition to store is defined to prepend as i + rev
it stores as 'iV'
similarly in the next iteration it gets 's'
and store as 'siV'
and so on.
Use a loop to find the factorial of a number.
num = 5
fac = 1
for i in range(1,num+1):
fac*=i
print(fac)
Output
120
Generate the Fibonacci series up to 10 terms using a while loop
# Initialize first two terms
a, b = 0, 1
# Number of terms to generate
count = 0
n = 10 # Total terms
while count <= n:
print(a, end=", ") # Print the current term
a, b = b, a + b # Update values for the next iteration
count += 1
Output
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55,
Use a for loop to check if a number is prime.
num = int(input('Enter a number: '))
if num <= 1:
print(f'{num} is not prime.')
else:
for i in range(2,num):
if num % i == 0:
print(f'{num} is not prime.')
break
else:
print(f'{num} is prime.')
We hope this article was helpful for you and you learned a lot about data analyst interview from it. If you have friends or family members who would find it helpful, please share it to them or on social media.
Join our social media for more.
Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your Understanding
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.