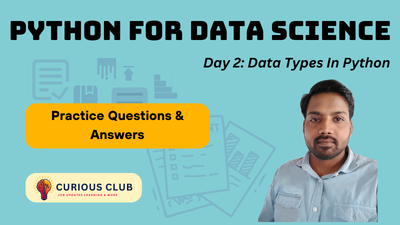
Test your Python skills with these 20 practice questions and solutions from Day 2 of Learning Python for Data Science. Covers string operations, data types, and more!
1. What will be the output of the following code?
x = "123"
y = int(x)
print(type(y))
Solution:
# Output: <class 'int'>
2. Extract the substring “learn” from the given string:
sentence = "Let's learn Python!"
Solution:
substring = sentence[6:11]
print(substring) # Output: 'learn'
3. Convert the float 7.5
into an integer and print the result.
Solution:
num = 7.5
int_num = int(num)
print(int_num) # Output: 7
4. Write a Python program to convert “Hello World” to uppercase.
Solution:
text = "Hello World"
print(text.upper()) # Output: 'HELLO WORLD'
5. What is the output of the following code?
x = "Data"
y = "Science"
print(x + y)
Solution:
# Output: 'DataScience'
6. Find the length of the string “Python Programming” using a built-in function.
Solution:
text = "Python Programming"
print(len(text)) # Output: 18
7. Write a Python code to extract “Science” from the string “Data Science”.
Solution:
text = "Data Science"
print(text[5:]) # Output: 'Science'
8. What will be the result of "Python"[::-1]
?
Solution:
print("Python"[::-1]) # Output: 'nohtyP'
9. How can you check if a string starts with a specific letter? (Use an example)
Solution:
text = "Python Programming"
print(text.startswith("P")) # Output: True
10. Replace all occurrences of “a” with “o” in the string “Data Analysis”.
Solution:
text = "Data Analysis"
print(text.replace("a", "o")) # Output: 'Doto Anolysis'
11. Convert the string “PYTHON” to lowercase.
Solution:
text = "PYTHON"
print(text.lower()) # Output: 'python'
12. What will be the output of "Hello" * 3
?
Solution:
print("Hello" * 3) # Output: 'HelloHelloHello'
13. Extract the last 3 characters from the string “Data Science”.
Solution:
text = "Data Science"
print(text[-3:]) # Output: 'nce'
14. Given a string text = " Machine Learning "
, write a Python command to remove leading and trailing spaces.
Solution:
text = " Machine Learning "
print(text.strip()) # Output: 'Machine Learning'
15. Convert the integer 2024
into a string.
Solution:
num = 2024
print(str(num)) # Output: '2024'
16. What function is used to determine the data type of a variable? Provide an example.
Solution:
num = 10
print(type(num)) # Output: <class 'int'>
17. Write a Python program to check whether a given string is empty or not.
Solution:
text = ""
print(len(text) == 0) # Output: True
18. What is the output of print("5" + "5")
?
Solution:
# Output: '55' (String concatenation, not addition)
19. Given a variable a = "Python"
, write a command to extract the first three characters.
Solution:
a = "Python"
print(a[:3]) # Output: 'Pyt'
20. What is the difference between .lower()
and .casefold()
in Python strings?
Solution: .lower()
converts a string to lowercase but .casefold()
is more aggressive as it is designed for case-insensitive string matching, handling special cases for certain languages.
Example:
text = "ß"
print(text.lower()) # Output: 'ß'
print(text.casefold()) # Output: 'ss'
Conclusion
These practice questions cover Python string manipulation, type conversions, and essential built-in functions. Practicing them will help solidify your understanding of these fundamental concepts.
We hope this article was helpful for you and you learned a lot about data analyst interview from it. If you have friends or family members who would find it helpful, please share it to them or on social media.
Join our social media for more.
Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your UnderstandingÂ
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.