Boost your Python skills with these practice questions and answers from Day 1 of learning Python for Data Science. Perfect for beginners looking to master Python basics! Read more
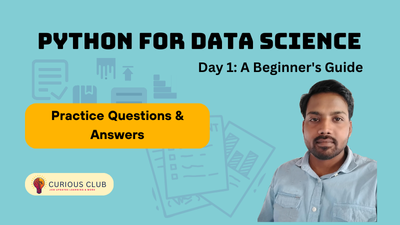
Practice Questions and Answers
These topics were covered in Day 1 article It is important to practice your learning in order to solidify your knowledge.
- What are some key fields where Python is widely used?
- Python is widely used in Data Analytics, Machine Learning, Web development, Cybersecurity, Game Development, and Finance, etc.
- Why is Python considered an easy-to-learn language?
- Python is considered easy to learn because of its clean and readable syntax, which resembles English language. Unlike other programming languages, Python does not require complex syntax structures.
- What makes Python different from compiled languages?
- Python is different from compiled language because it is an interpreted language, meaning the code is executed line by line rather than being compiled into machine code first. This allows for a quick testing and debugging.
- What does it mean when we say Python is dynamically typed?
- Python is dynamically typed, meaning you do not need to declare a variable type explicitly. The interpreter automatically determines the data type at runtime based on the assigned value.
- Name two Python libraries commonly used in data science.
- NumPy and Pandas are two commonly used libraries in data science.
- NumPy (Numerical Python) is used for numerical computations, supporting large multidimensional arrays and mathematical operations.
- Pandas is used for data manipulation and analysis. providing powerful data structures like DataFrames for handling structured data efficiently.
- NumPy and Pandas are two commonly used libraries in data science.
- Why is Python considered cross-platform?
- Python is considered cross-platform because it can run on multiple operating systems, including Windows, macOS, and Linux, without requiring changes to the code. This is possible because Python interpreter handles system-specific differences.
- How does Python’s interpreted nature help in debugging?
- Python’s interpreted nature helps in debugging because code is executed line by line, stopping immediately when an error occurs. Additionally, Python provides descriptive error messages, making debugging more efficient.
- How do you assign a value to a variable in Python? Provide an example.
site = 'CuriousCLub.in'
description ='CuriousClub.in offers extensive learning resources on Python, SQL, Data Visualization, Excel, and job alerts to help users upskill and find career opportunities.'
number_of_article = 1000
- What are the rules for naming variables in Python?
- Variable names in Python must start with a letter (a-z, A-Z) or an underscore (_) but cannot start with a number, it cannot contain a space or special characters (e.g.,
@, $, %
) and since Python is a case sensitive languagename
andName
are considered as two different variables.
- Variable names in Python must start with a letter (a-z, A-Z) or an underscore (_) but cannot start with a number, it cannot contain a space or special characters (e.g.,
- Is Python case-sensitive when it comes to variable names? Explain with an example.
- Yes, Python is a case sensitive language, for example,
name = 'your name'
Name = 'please provide your full name'
print(name) # Output: your name
print(Name) # Output: please provide your full name
Since Python distinguishes between uppercase and lowercase letters, changing the capitalization of a variable name creates a new, separate variable.
- What happens if you assign
x = 10
in Python without declaring a type?- If you assign
x = 10
in without declaring a type, Python automatically determines the data type based on the assigned value. Since10
is a whole number,x
will be assigned the integer (int
) type.
- If you assign
x = 10
print(type(x)) # Output: <class 'int'>
- What is the output of the following code?
- a = 5
- b = 10
- a, b = b, a
- print(a, b)
This code swaps the values of a
and b
, so the output will be:
10, 5
- What is the purpose of comments in Python?
- Comments are used to add notes or explanations to codes without affecting its execution. They help make the code more readable and understandable, especially for complex logic or when working in a team.
- How do you write a single-line comment in Python?
- Single line comments start with # symbol and continue to end of the line.
- How do you write a multi-line comment in Python?
- Multiline comments are written inside triple quotes (”’ or “””).
- What is the difference between single-line and multi-line comments?
- Single-line comments in Python start with the
#
symbol and are used to add brief explanations within the code.
- Single-line comments in Python start with the
# This is a single-line comment
x = 10 # Assigning value to x
-
- Multi-line comments are written using triple quotes (
'''
or"""
) and are used for longer explanations or documentation.
- Multi-line comments are written using triple quotes (
"""
This is a multi-line comment.
It can span multiple lines.
"""
print("Hello, World!")
- How can comments help in debugging?
- Temporarily Disabling Code – You can comment out specific lines to test different parts of your code without deleting them.
- Explaining Complex Logic – Adding comments helps in understanding intricate logic, making it easier to debug later.
- Tracking Issues – Comments can mark potential problem areas or suggest improvements.
Temporarily Disabling Code
x = 10
# y = 20 # Disabling this line to check for errors
print(x)
Explaining Complex Logic
# Checking if the number is even or odd
if x % 2 == 0:
print("Even")
else:
print("Odd")
Tracking Issues
# TODO: Optimize this loop for better performance
for i in range(1000):
print(i)
- What is the significance of using
#
in Python?- The
#
symbol in Python is used to create single-line comments. Anything written after#
on the same line is ignored by the Python interpreter and does not affect code execution.
- The
- Can a variable name in Python start with a number? Why or why not?
- No, a variable name in Python cannot start with a number because Python requires variable names to begin with a letter or an underscore. Starting with a number results in a SyntaxError.
- What is the output of the following code?
# Assigning values
x = "Python"
y = 3.14
print(x, y)
Python 3.14
The print(x, y)
statement prints the values of x
and y
, separated by a space.
- Write a Python program to print “Welcome to Python Programming!” using the
print()
function.- print(‘Welcome to Python Programming!’)
- Create three variables:
name
,age
, andcity
. Assign them your name, age, and city respectively. Then, use theprint()
function to display their values in a sentence.
name = 'Vishal'
age = 27
city = 'Delhi'
print(f'My name is {name}, i am {age} years old, I am from {city}.')
- Create two variables
x
andy
. Assign any two numbers to them. Calculate their sum, difference, product, and quotient. Print each result.
x, y = 5, 10
print('Sum of x and y is :',x+y)
print('Difference of x and y is :',x-y)
print('Product of x and y is :',x*y)
print('Qoutient of x and y is :',x/y)
Sum of x and y is : 15
Difference of x and y is : -5
Product of x and y is : 50
Quotient of x and y is : 0.5
- Write a Python program to swap the values of two variables without using a third variable. Example: If
a = 3
andb = 5
, after swappinga
should be 5 andb
should be 3.
a = 3
b = 5
a, b = b, a
print(a)
print(b)
5
3
- Write a Python script that includes both single-line and multi-line comments.
# this is a single line of comment.
'''
This is a multiline comment.
line 2 of comment.
'''
- Variables and Assignment: Create a variable language and assign it the value “Python”. Print the variable.
language = 'Python'
print(language)
- Basic Data Types: Declare a variable for each of the following data types: integer, float, and string. Print their values.
integer = 58
float_ = 34.34
string = 'string'
print(integer)
print(float_)
print(string)
58
34.34
string
- Dynamic Type Change: Assign the value 10 to a variable and print its type. Change its value to 10.5 and print its type again. Finally, assign “Ten” to the same variable and print its type. Explain why Python allows this.
variable = 10
print(type(variable))
variable = 10.5
print(type(variable))
variable = 'Ten'
print(type(variable))
<class 'int'>
<class 'float'>
<class 'str'>
- Write a single line of code to assign the values “Apple”, 20, and 3.14 to three different variables. Print all three variables in a single print() statement, ensuring they are separated by ” | “.
fruit, price, weight = "Apple", 20, 3.14
print(fruit,"|", price,"|", weight )
Apple | 20 | 3.14
This concludes Chapter 1. In the next chapter, we will explore Python’s data types and operations in detail.
You can also check out our podcast on this topic:
Practice Questions and Answers Practice Questions and Answers Practice Questions and Answers Practice Questions and Answers Practice Questions and Answers Practice Questions and Answers Practice Questions and Answers Practice Questions and Answers Practice Questions and Answers Practice Questions and Answers Practice Questions and Answers
We hope this article about Practice questions for day 1 of Learning Python for Data Science was helpful for you and you learned a lot of new things from it. If you have friends or family members who would find it helpful, please share it to them or on social media.
Join our social media for more.
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your Understanding
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.