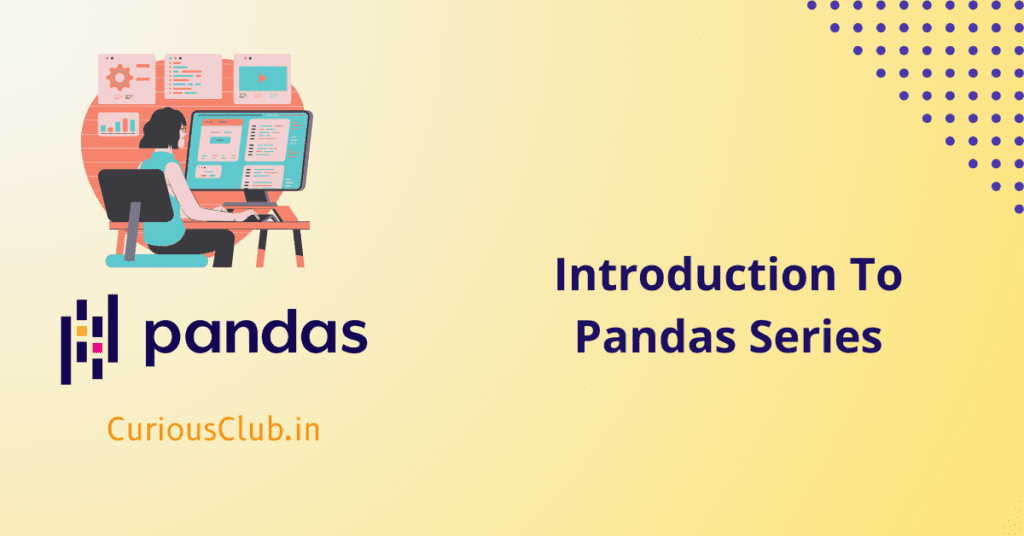
Dominate Data Wrangling with Pandas Series!
Pandas, a cornerstone library in Python’s data science arsenal, offers a treasure trove of tools for data manipulation and analysis. At its core lies the Series, a one-dimensional powerhouse capable of storing and manipulating data of various types. This guide empowers you to grasp the essence of Pandas Series, unlocking its potential for efficient data handling in your Python projects.
What is a Pandas Series?
Imagine a single, flexible column in a spreadsheet – that’s the essence of a Pandas Series! It’s a one-dimensional labeled array, meaning each data point has a corresponding label (index) for effortless retrieval and organization. This structure excels in handling various data types, including numbers, text, and booleans, making it a versatile tool for diverse data wrangling tasks.
Here’s a table to illustrate the concept:
Index (Labels) | Data |
---|---|
Fruit 1 | Apple |
Fruit 2 | Banana |
Fruit 3 | Cherry |
Fruit 4 | Mango |
This table represents a Series with fruits as data and custom labels (“Fruit 1”, “Fruit 2”, etc.) as the index. You can access specific fruits using their corresponding labels or positions.
Key Features of Pandas Series
- Flexible Data Types: Handles various data types (numbers, text, booleans, etc.).
- Intuitive Indexing: Access data by labels or positions.
- Powerful Operations: Filter, sort, aggregate, and more on your data.
Why Use Pandas Series?
- Efficient Data Handling: Series simplifies data cleaning, transformation, and analysis.
- Works with DataFrames: Integrates with DataFrames for complex data models.
- Data Analysis Powerhouse: Series is foundational for advanced data analysis in Python.
Creating Pandas Series
Using Lists
import pandas as pd
data = [1, 5, 8, 2, 4]
my_series = pd.Series(data)
print(my_series)
- We import the pandas library as
pd
for convenience. - A list
data
is created containing numerical values. - The
pd.Series(data)
function creates a Series from the listdata
. print(my_series)
displays the newly created Series.
0 1
1 5
2 8
3 2
4 4
dtype: int64
- The output shows each data point from the original list along with a corresponding index.
- The index starts from 0 (zero-based indexing) and increments by 1 for each subsequent element in the Series.
- The
dtype: int64
at the end indicates the data type of the elements in the Series, which in this case is integer (int64).
Using Dictionaries
data = {"apple": 10, "banana": 15, "cherry": 20}
my_series = pd.Series(data)
print(my_series)
- A dictionary
data
is created with keys representing fruit names and values representing their prices. - The
pd.Series(data)
function creates a Pandas Series from the dictionarydata
. - Custom labels (index) are not explicitly set in this example, so the dictionary keys become the default index.
print(my_series)
displays the Series with fruit names as index and their corresponding prices as data.
apple 10
banana 15
cherry 20
dtype: int64
- Each dictionary key becomes the index label for the corresponding value in the Series.
- The values from the dictionary become the data points in the Series.
dtype: int64
indicates the data type of the Series elements (integers in this case).
Setting Custom Index
data = ["apple", "banana", "cherry", "mango"]
labels = ["Fruit 1", "Fruit 2", "Fruit 3", "Fruit 4"]
my_series = pd.Series(data, index=labels)
print(my_series)
- A list
data
is created containing fruit names. - A separate list
labels
is created with custom labels for each fruit. - The
pd.Series(data, index=labels)
function creates a Series from the listdata
and assigns the custom labels fromlabels
as the index. print(my_series)
displays the Series with custom labels (“Fruit 1”, “Fruit 2”, etc.) as the index and fruit names as data.
Fruit 1 apple
Fruit 2 banana
Fruit 3 cherry
Fruit 4 mango
dtype: object
- Fruit 1: This is the custom label (index) from the
labels
list. - apple: This is the corresponding data value from the
data
list at the same position (0th index) as the “Fruit 1” label. - Similarly, “Fruit 2” is paired with “banana”, “Fruit 3” with “cherry”, and “Fruit 4” with “mango”.
dtype: object
: This indicates that the data type of the Series elements is ‘object’, which means it can hold various data types like strings in this case.
Accessing Elements in a Series:
Using Index Labels:
my_series = pd.Series(["apple", "banana", "cherry", "mango"], index=["Fruit 1", "Fruit 2", "Fruit 3", "Fruit 4"])
fruit_at_index_2 = my_series["Fruit 2"]
print(fruit_at_index_2)
- A Series
my_series
is created with a list of fruits (["apple", "banana", "cherry", "mango"]
) and custom labels (index) as a separate list (["Fruit 1", "Fruit 2", "Fruit 3", "Fruit 4"]
). - To access a specific element by its index label, we use square brackets
[]
with the desired label name inside. In this case,my_series["Fruit 2"]
retrieves the element associated with the label “Fruit 2”.
banana
- The output (
banana
) confirms that we successfully accessed the element with the label “Fruit 2”, which is “banana” in this Series.
Using Positional Indexing (Integer-Based):
first_fruit = my_series[0]
print(first_fruit)
apple
- In Python, indexing starts from 0. So,
fruits[0]
retrieves the element at index 0, which is “apple” in our case.
Performing Basic Logical Operations:
Comparison Operators:
You can use comparison operators like ==
, !=
, <
, >
, <=
, and >=
to create boolean Series based on conditions.
prices = pd.Series([5, 12, 8, 15, 9])
expensive_items = prices > 10
print(expensive_items)
0 False
1 True
2 False
3 True
4 False
dtype: bool
- The code creates a Series of prices (
prices
). - It then creates a new Series (
expensive_items
) that identifies which prices in the original Series are greater than 10 using a boolean comparison. - Finally, it prints the resulting Series (
expensive_items
), showing True for expensive items and False for non-expensive items.
Boolean Operators:
You can use boolean operators like &
(AND), |
(OR), and ~
(NOT) to combine boolean Series.
prices = pd.Series([12, 5, 18, 9, 15])
in_stock = pd.Series([True, False, True, False, True])
expensive_items = prices > 10
available_expensive_items = (expensive_items & in_stock)
print(available_expensive_items)
0 True
2 True
4 True
dtype: bool
- Find Expensive Items: The
expensive_items
Series holds True for items with prices greater than 10. - Find Available Expensive Items: We combine
expensive_items
andin_stock
using the&
operator. This ensures only items that are both expensive AND in stock are marked as True inavailable_expensive_items
. - Output: The
print
statement shows which items are both expensive and available for purchase.
Reference:
By understanding Pandas Series and their functionalities, you’ve unlocked a powerful tool for data manipulation and analysis in Python. You can leverage Series for tasks like filtering, sorting, and performing calculations on your data with ease.
Feel free to share your thoughts and questions in the comments below! We hope that you liked our information, if you liked our information, then you must share it with your friends, family and group. So that they can also get this information.
Also Read:
- Pandas Series: Unleash the Power of Data
- NumPy Delete
- NumPy Insert
- NumPy Append
- Introduction to Pandas
- Introduction to NumPy
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.