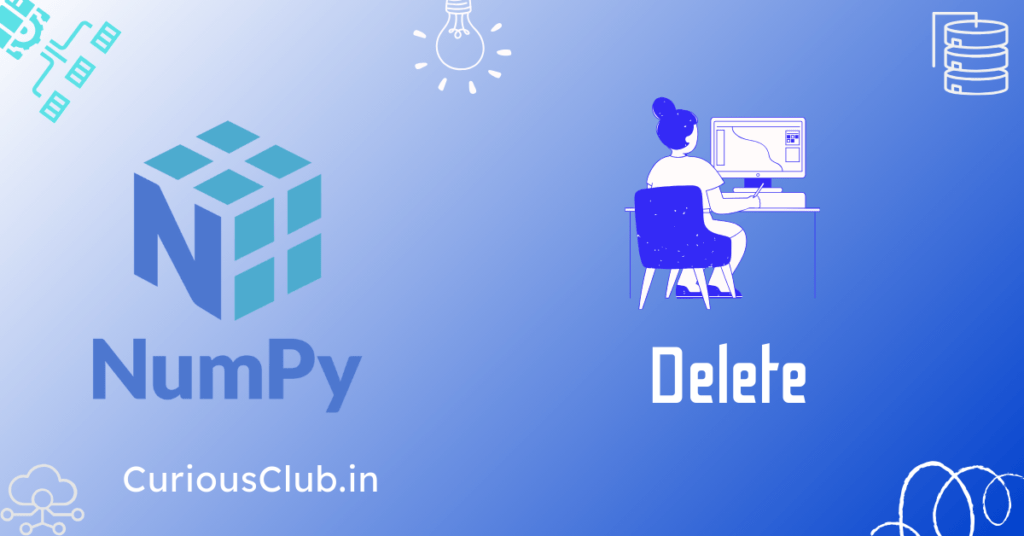
In this article we will learn about NumPy Delete functions, we will explore their usage, benefits, and best practices, along with examples.
What does NumPy Delete function do?
Deletion involves removing elements or entire sections from an array, thereby reducing its size. It facilitates data cleanup, filtering, and optimization of array structures.
NumPy Delete
np.delete()
:- The
np.delete()
function removes specified elements or slices from an array along a given axis. - It facilitates selective data removal, reshaping, and array optimization.
- The
- Syntax
- numpy.delete(arr, obj, axis=None)
- arr: This is the input array from which elements will be deleted.
- obj: This specifies the index or indices indicating which elements should be deleted. It can be:
- An integer: If obj is an integer, the element at that index is deleted.
- A slice object: If obj is a slice object, the elements indicated by the slice are deleted.
- An array-like: If obj is an array-like object, the elements indicated by the array-like object are deleted.
- axis: This is the axis along which the deletion should be performed. By default, axis is None, and the input array is flattened before the deletion. If axis is an integer, elements are deleted along the specified axis. If axis is None, elements are removed from the flattened input array.
- numpy.delete(arr, obj, axis=None)
Benefits and Applications of Deletion
- Array Optimization:
- Deletion enables users to remove unnecessary elements or arrays from arrays, optimizing memory usage and computational performance.
- It streamlines data cleanup and filtering processes, enhancing array readability and maintainability.
Best Practices for Deletion
- Memory Efficiency:
- Use NumPy’s array views and slicing capabilities to minimize memory overhead during deletion operations.
- Handle edge cases gracefully to prevent errors and maintain array integrity.
Practical Example for Deletion
1D array
arr = np.array([1, 2, 3, 4, 5])
deleted_arr = np.delete(arr, [1, 3])
print(deleted_arr)
- We use the
np.delete()
function to delete the elements at indices[1, 3]
from the arrayarr
. - The resulting array
deleted_arr
contains the elements ofarr
with the elements at indices[1, 3]
removed.
[1 3 5]
- This output shows the modified array
deleted_arr
after the deletion operation. The elements at indices[1, 3]
(i.e.,2
and4
) have been removed from the original arrayarr
.
2D array
axis = 0
arr = np.array([[1, 2, 3], [4, 5,6],[7,8,9]])
new_arr = np.delete(arr, 1, axis = 0)
print(new_arr)
- We use the
np.delete()
function to delete the row at index1
along axis0
(rows) from the arrayarr
.
[[1 2 3]
[7 8 9]]
- This output shows the modified array
new_arr
after the deletion operation. The row at index1
(i.e.,[4, 5, 6]
) has been removed from the original arrayarr
.
axis = 1
arr = np.array([[1, 2, 3], [4, 5,6],[7,8,9]])
new_arr = np.delete(arr, 1, axis = 1)
print(new_arr)
- We use the
np.delete()
function to delete the column at index1
along axis1
(columns) from the arrayarr
.
[[1 3]
[4 6]
[7 9]]
- This output shows the modified array
new_arr
after the deletion operation. The column at index1
(i.e.,[2, 5, 8]
) has been removed from the original arrayarr
.
In conclusion, the numpy.delete()
function offers a versatile solution for manipulating NumPy arrays, enabling users to efficiently remove specific elements or sub-arrays along designated axes. By mastering its syntax and capabilities, individuals can effectively manage and transform their data arrays, enhancing their data analysis workflows. As you explore the diverse applications of numpy.delete()
, consider sharing your newfound knowledge with others in the data science community. Sharing educational articles or tutorials on platforms like blogs, forums, or social media can contribute to the collective learning experience and foster collaboration among peers. Together, we can continue to expand our understanding of NumPy and its powerful array manipulation capabilities.
We hope that you liked our information, if you liked our information, then you must share it with your friends, family and group. So that they can also get this information.
Reference: np.delete()
Also Read:
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.