NumPy, the powerhouse of numerical computing in Python, empowers users with an array of functions for efficient data manipulation. Among its arsenal lies the versatile tool of concatenation, allowing for seamless merging of arrays along specified axes. In this article, we delve into NumPy concatenation, exploring its functionalities, applications, and best practices.
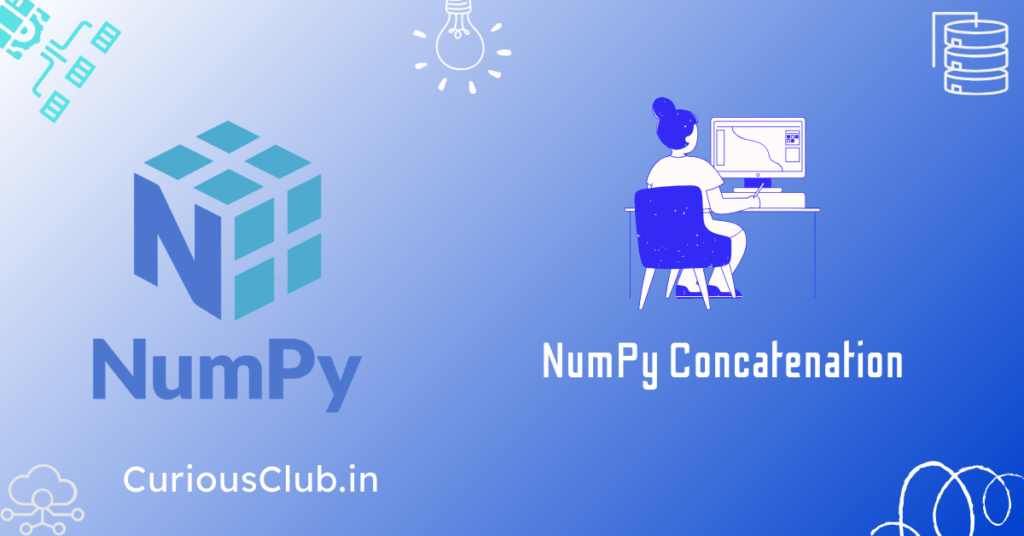
Understanding NumPy Concatenation
Concatenation:
- Concatenation is the process of combining arrays along specified axes to form a larger array.
- It enables users to merge arrays both horizontally and vertically, expanding their dimensions as needed.
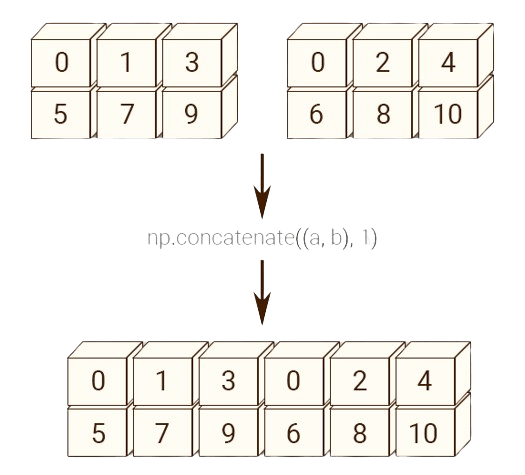
Functions for Concatenation
np.concatenate()
:- The
np.concatenate()
function concatenates arrays along a specified axis. - It offers flexibility in combining arrays of different shapes, provided they have compatible dimensions along the concatenation axis.
- The
np.vstack()
andnp.hstack()
:np.vstack()
stacks arrays vertically, i.e., along the first axis (axis=0), to increase the number of rows.np.hstack()
stacks arrays horizontally, i.e., along the second axis (axis=1), to increase the number of columns.
Benefits and Applications
Data Integration:
- Concatenation facilitates the integration of datasets with complementary attributes but matching dimensions along the concatenation axis.
- It streamlines the process of combining data from multiple sources into a single, cohesive dataset.
Array Manipulation:
- Concatenation enables users to construct larger arrays by combining smaller arrays along specified axes.
- It simplifies tasks such as extending arrays, aggregating data, and creating multi-dimensional structures.
Best Practices
Axis Specification
- Always specify the axis along which concatenation should occur to ensure the desired outcome.
- Understand the dimensions of your arrays and how they align along the concatenation axis.
Input Validation:
- Validate the dimensions of input arrays to ensure compatibility for concatenation.
- Handle edge cases gracefully to prevent errors during concatenation.
Practical Examples
Concatenated Array along axis 0 (rows):
arr1 = np.array([[1, 2], [3, 4]])
arr2 = np.array([[5, 6, 7], [8, 9, 10]])
concatenated_arr = np.concatenate((arr1, arr2), axis=0)
print("Concatenated Array along axis 0:")
print(concatenated_arr)
[[ 1 2]
[ 3 4]
[ 5 6 7]
[ 8 9 10]]
In this example, arr1
has a shape of (2, 2) and arr2
has a shape of (2, 3). By concatenating along axis 0, the arrays are stacked vertically, resulting in a new array with a shape of (4, 3).
Concatenate along axis 1 (columns)
arr1 = np.array([[1, 2], [3, 4]])
arr2 = np.array([[5, 6], [7, 8]])
concatenated_arr = np.concatenate((arr1, arr2), axis=1)
print(concatenated_arr)
[[1 2 5 6]
[3 4 7 8]]
In this example:
- Two arrays,
arr1
andarr2
, with the same number of rows are created. - The
np.concatenate()
function is used to concatenate the arrays along axis 1 (columns). - The resulting
concatenated_arr
contains the elements ofarr1
followed by the elements ofarr2
, forming a new array with an increased number of columns.
For details of hstack and vstack click here
Conclusion
NumPy concatenation is a powerful tool for efficiently merging arrays in Python. By understanding its functionalities and applications, users can streamline data integration tasks, manipulate arrays with ease, and unlock new possibilities in numerical computing. Incorporate NumPy concatenation into your workflows to enhance productivity and accelerate data-driven insights.
We hope that you liked our information, if you liked our information, then you must share it with your friends, family and group. So that they can also get this information.
Also Read:
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.