Understanding how to efficiently manipulate data in Python is crucial, and NumPy‘s array indexing and slicing features are indispensable tools in this regard. Here’s a concise overview to help you master these essential NumPy techniques:
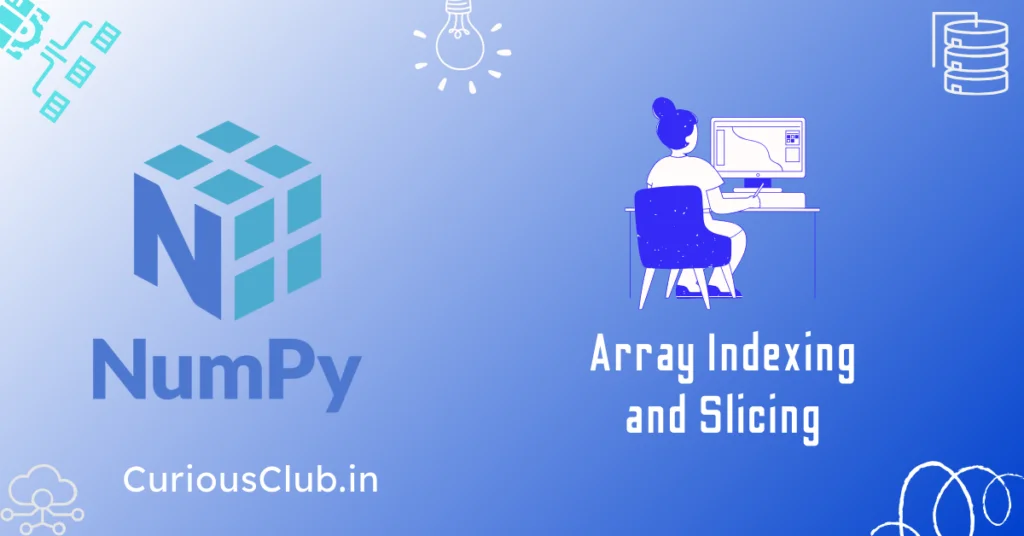
Basic Techniques
Basic Indexing:
- In NumPy, array elements can be accessed using square brackets
[]
and zero-based indices. - Individual elements are accessed by specifying their indices within square brackets, similar to standard Python lists.
import numpy as np
# Create a simple 1D array
arr_1d = np.array([1, 2, 3, 4, 5])
# Access individual elements
print(arr_1d[0]) # Output: 1
print(arr_1d[2]) # Output: 3
# Negative indices count from the end of the array
print(arr_1d[-1]) # Output: 5 (last element)
# Create a 2D array
arr_2d = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
# Access individual elements
print(arr_2d[0, 0]) # Output: 1 (first row, first column)
print(arr_2d[1, 2]) # Output: 6 (second row, third column)
In these examples, we create both 1D and 2D arrays using NumPy. Then, we access individual elements of the arrays using square brackets and zero-based indices.
Slicing:
- NumPy arrays support slicing, allowing you to extract subarrays or contiguous segments of an array.
- Slicing syntax follows the format
[start:stop:step]
, wherestart
is the starting index,stop
is the ending index (exclusive), andstep
is the stride.
# Create a simple 1D array
arr_1d = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
# Extract a subarray from index 2 to index 5 (exclusive)
print(arr_1d[2:5]) # Output: [3 4 5]
# Extract elements starting from index 0 to index 8 (exclusive) with a step of 2
print(arr_1d[0:8:2]) # Output: [1 3 5 7]
# Reverse the array
print(arr_1d[::-1]) # Output: [10 9 8 7 6 5 4 3 2 1]
# Create a 2D array
arr_2d = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
# Extract a subarray consisting of the first two rows and all columns
print(arr_2d[:2, :]) # Output: [[1 2 3]
[4 5 6]]
# Extract a subarray consisting of all rows and the last two columns
print(arr_2d[:, -2:]) # Output: [[2 3]
[5 6]
[8 9]]
Advanced Techniques
Boolean Indexing:
- Boolean indexing involves selecting elements based on a conditional expression, where only elements that satisfy the condition are included in the result.
# Create an array
arr = np.array([1, 2, 3, 4, 5])
# Define a condition: select elements greater than 2
condition = arr > 2
# Apply boolean indexing
selected_elements = arr[condition]
print(selected_elements) # Output: [3 4 5]
In this example, we create a NumPy array arr
containing values from 1 to 5. We then define a condition arr > 2
, which evaluates to a boolean array where True
indicates elements greater than 2 and False
indicates elements less than or equal to 2. Finally, we use boolean indexing with the condition to select elements greater than 2 from the original array arr
, resulting in [3, 4, 5]
.
- Boolean arrays (arrays of
True
andFalse
values) are used to filter elements from the original array.
# Create an array
arr = np.array([1, 2, 3, 4, 5])
# Define a boolean condition
condition = np.array([True, False, True, False, True])
# Use boolean indexing to select elements based on the condition
selected_elements = arr[condition]
print(selected_elements) # Output: [1 3 5]
In this example, we create a boolean array condition
with True
and False
values. We then use boolean indexing with this condition to select elements from the original array arr
. Elements corresponding to True
values in the condition array are selected, resulting in [1, 3, 5]
.
Indexing
- Integer indexing allows you to select specific elements from an array by specifying their indices as an array of integers.
# Create an array
arr = np.array([1, 2, 3, 4, 5])
# Define indices to select specific elements
indices = np.array([0, 2, 4])
# Use integer indexing to select elements based on the indices
selected_elements = arr[indices]
print(selected_elements) # Output: [1 3 5]
In this example, we have an array arr
containing elements from 1 to 5. We define an array indices
containing the indices of the elements we want to select (0
, 2
, and 4
). We then use integer indexing with these indices to select the corresponding elements from the original array arr
, resulting in [1, 3, 5]
.
- This technique is particularly useful for non-contiguous selection of elements or rearranging the order of elements in an array.
# Create an array
arr = np.array([1, 2, 3, 4, 5])
# Define indices to select specific elements in a non-contiguous manner
indices = np.array([4, 2, 0])
# Use integer indexing to select elements based on the indices
selected_elements = arr[indices]
print(selected_elements) # Output: [5 3 1]
In this example, we have an array arr
containing elements from 1 to 5. We define an array indices
containing the indices of the elements we want to select (4
, 2
, and 0
). We then use integer indexing with these indices to select the corresponding elements from the original array arr
, resulting in [5, 3, 1]
. This demonstrates how integer indexing can be used for non-contiguous selection of elements or rearranging the order of elements in a NumPy array.
Exploring Array Slicing
NumPy array slicing enables you to extract portions of an array along one or more dimensions. It offers flexibility in selecting subsets of data without copying the original array, making it efficient for handling large datasets.
Single-Dimensional Slicing:
- In a one-dimensional array, slicing is straightforward and follows the
[start:stop:step]
syntax.
# Create a one-dimensional array
arr = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
# Slice the array to get elements from index 2 to index 7 (exclusive)
sliced_arr = arr[2:7]
print(sliced_arr) # Output: [3 4 5 6 7]
In this example, we have a one-dimensional array arr
containing elements from 1 to 10. We use slicing with the [start:stop]
syntax to select elements from index 2 to index 7 (exclusive), resulting in the subarray [3, 4, 5, 6, 7]
.
- Omitting
start
orstop
defaults to the beginning or end of the array, respectively.
# Create a one-dimensional array
arr = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
# Slice the array to get elements from the beginning up to index 5
sliced_arr_beginning = arr[:5]
print(sliced_arr_beginning) # Output: [1 2 3 4 5]
# Slice the array to get elements from index 5 to the end
sliced_arr_end = arr[5:]
print(sliced_arr_end) # Output: [ 6 7 8 9 10]
In these examples, omitting the start
parameter defaults to the beginning of the array, while omitting the stop
parameter defaults to the end of the array.
Multi-Dimensional Slicing:
- For multi-dimensional arrays, slicing is performed along each dimension separately, separated by commas.
arr[ start_row : end_row , start_column : end_column]
# Create a two-dimensional array
arr_2d = np.array([[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]])
# Slice the array to get the first two rows and all columns
sliced_arr_rows = arr_2d[:2, :]
print(sliced_arr_rows)
# Output: [[1 2 3 4]
[5 6 7 8]]
# Slice the array to get all rows and the last two columns
sliced_arr_columns = arr_2d[:, -2:]
print(sliced_arr_columns)
# Output: [[ 3 4]
[ 7 8]
[11 12]]
In this example, we have a two-dimensional array arr_2d
. We use slicing to extract subsets of the array:
sliced_arr_rows
contains the first two rows and all columns ofarr_2d
.sliced_arr_columns
contains all rows and the last two columns ofarr_2d
.
- Slices can be specified independently for each dimension, allowing for complex selections of subarrays.
# Create a two-dimensional array
arr_2d = np.array([[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]])
# Slice the array to get a subarray with rows from index 1 to index 3 (exclusive) and columns from index 1 to index 3 (exclusive)
subarray = arr_2d[1:3, 1:3]
print(subarray)
# Output: [[ 6 7]
[10 11]]
In this example, we have a two-dimensional array arr_2d
. We use slicing to extract a subarray:
- The slice
[1:3]
specifies rows from index 1 to index 3 (exclusive), which selects rows 1 and 2. - The slice
[1:3]
specifies columns from index 1 to index 3 (exclusive), which selects columns 1 and 2. - Together, these slices select the subarray
[[6, 7], [10, 11]]
.
Best Practices for Efficiency in indexing and slicing
- Embrace vectorized operations for faster data processing.
- Be mindful of memory usage, particularly with large datasets.
- Understand array broadcasting principles for efficient element-wise operations.
Conclusion
Unlocking the potential of NumPy array indexing and slicing is essential for proficient data manipulation in Python. Experiment with different techniques to streamline your workflows and unleash the full power of NumPy arrays for your data analysis tasks.
We hope that you liked our information, if you liked our information, then you must share it with your friends, family and group. So that they can also get this information.
Also Read:
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.