NumPy is used to work on arrays. The array object is also referred as ndarray. There are multiple mechanisms about how to create array.
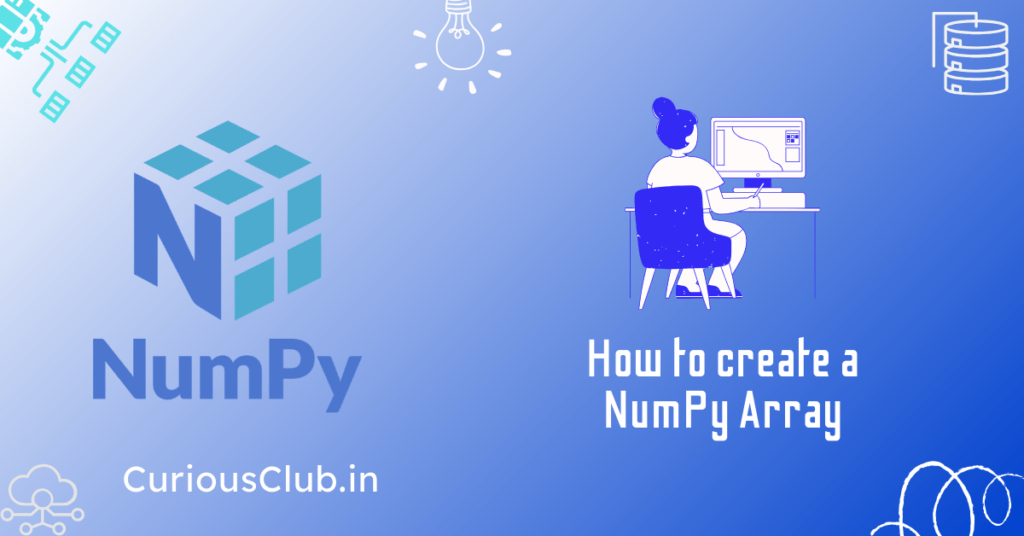
Conversion from other Python structures
NumPy arrays can be defined using other Python sequences such as lists and tuples. List are defined using [….] and Tuples are defined using (……)
a list will create a 1D array,
a1D = np.array([1,2,3,4,5])
a list of lists will create a 2D array,
a2D = np.array([[1,2,3], [4,5,6]])
nested list will create higher dimensional arrays.
a3D = np.array([[[1,2], [3,4]], [{5,6], [7,8]]]
to learn about dimension in NumPy arrays, you can refer Introduction to NumPy | NumPy Array | NumPy – CuriousClub
Intrinsic NumPy array creation functions
- 1D arrays
- 2D arrays
- ndarrays
There are over 40 functions to create arrays, as documented in array creation routines. these functions can be divided into three categories based on dimension.
1D array creation
1D array creation function numpy.linspace and numpy.arage generally need at least two inputs, start and stop.
numpy.arrage creates arrays with regularly incrementing values.
if only one value is passed to arrange it will be treated as stop value as in the below example an array with numbers from 0 to 9 has been created
arr = np.arange(10)
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
Alternatively, data type can be defined in arrage function when defined resulting array will be of that data type as in code below the data type has been defined to float, hence the resulting array contains only float values.
arr = np.arange(10, dtype=float)
array([0., 1., 2., 3., 4., 5., 6., 7., 8., 9.])
A start and stop value can also be defined in arrange function, when defined the start value is included but stop value will be excluded meaning it will not be part of the resulting array. In below example when range is defined as 1 to 10 resulting array contains numbers from 1 to 9.
arr = np.arange(1,10)
array([1, 2, 3, 4, 5, 6, 7, 8, 9])
When start, stop and step is defined it results in an array having values from start to stop with the specified step size.
arr = np.arange(2,3,0.1)
array([2. , 2.1, 2.2, 2.3, 2.4, 2.5, 2.6, 2.7, 2.8, 2.9])
2D array creation
The functions numpy.eye, numpy.diag, and numpy.vander are used to create 2D arrays with special properties. These arrays represent specific types of matrices, such as identity matrices, diagonal matrices, and Vandermonde matrices, respectively.
numpy.eye(n, m) – creates identity matrix.
np.eye(n, m)
is a NumPy function used to create a 2D array representing an identity matrix with dimensions n
by m
.
An identity matrix is a square matrix with ones on the main diagonal (from the top-left to the bottom-right) and zeros elsewhere. It has the property that when multiplied with any other matrix, it leaves the other matrix unchanged.
np.eye(3)
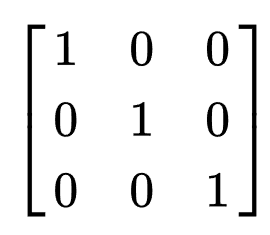
np.eye(3, 5)
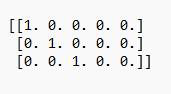
numpy.diag(values) – create square 2D arrays.
numpy.diag is a versatile function that can be used to create square 2D arrays with specified diagonal values or extract diagonal elements from existing arrays, making it valuable for linear algebra tasks.
numpy.diag([1, 2, 3])
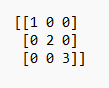
When used with a single argument, numpy.diag
creates a square 2D array with the given values along the main diagonal (from the top-left to the bottom-right) and zeros elsewhere.
np.diag([1, 2, 3], 1)
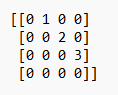
The np.diag([1, 2, 3], 1)
function call creates a 2D array with the provided values along a specified diagonal of the array.
numpy.vander(x, n) – creates vandermonde matrix
vander
function creates a matrix where each column contains powers of the input array x
, from highest to lowest order, up to the specified polynomial order n-1
. This matrix is commonly used in polynomial regression to fit a polynomial curve to a set of data points.
np.vander(np.linspace(0, 2, 5), 2)
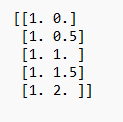
- np.linspace(0, 2, 5) generates an array of 5 equally spaced numbers between 0 and 2, inclusive. This array is [0., 0.5, 1., 1.5, 2.].
- np.vander(array, 2) constructs a Vandermonde matrix from the given array with a polynomial order of 2.
General ndarray creation functions
The functions for creating ndarrays, such as numpy.ones, numpy.zeros, and random, generate arrays based on specified shapes. By providing the desired dimensions and lengths along each dimension in a tuple or list, these functions can create arrays of any dimension.
numpy.zeros will create an array filled with 0 values with the specified shape.
np.zeros((2, 3))
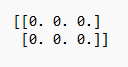
The code np.zeros((2, 3))
creates a NumPy array of shape 2×3 filled with zeros.
(2, 3)
specifies the shape of the array. In this case, it creates a 2-dimensional array with 2 rows and 3 columns.
np.zeros((2, 3, 2))
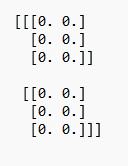
The code np.zeros((2, 3, 2))
creates a NumPy array of shape 2x3x2 filled with zeros.
(2, 3, 2)
specifies the shape of the array. In this case, it creates a 3-dimensional array with:- 2 layers,
- 3 rows in each layer,
- 2 columns in each row.
numpy.ones will create an array filled with 1 values.
np.ones((2, 3))
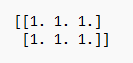
(2, 3)
specifies the shape of the array. In this case, it creates a 2-dimensional array with 2 rows and 3 columns.
np.ones((2, 3, 2))
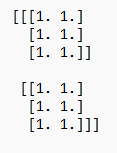
(2, 3, 2)
specifies the shape of the array. In this case, it creates a 3-dimensional array with:
- 2 layers,
- 3 rows in each layer,
- 2 columns in each row.
We hope that you liked our information, if you liked our information, then you must share it with your friends, family and group. So that they can also get this information.
Also Read:
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.