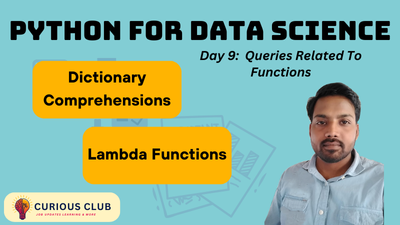
Welcome to Day 9 of Learning Python for Data Science. Today we will explore comprehensions, dictionary comprehensions, and lambda functions — powerful tools that help write cleaner and more concise code. These features enable efficient data transformation and manipulation, especially when working with large datasets. In this article, we’ll cover list and dictionary comprehensions, introduce lambda (anonymous) functions, and see how they are used in real-world data science applications.
Day 8 of Learning Python for Data Science – All About Functions In Python
List Comprehensions
Create a List of Squares from 1 to 10
lis = [i**2 for i in range(1, 11)]
print(lis)
Create a List of Numbers from 1 to 50 Divisible by 3 and 5
lis = [i for i in range(1, 51) if i % 3 == 0 and i % 5 == 0]
print(lis)
Given a Sentence, Create a List of Word Lengths
sentence = "Given a sentence, create a list of the length of each word in the sentence using list comprehension"
word_lengths = [len(word) for word in sentence.split()]
print(word_lengths)
Extract All Even Numbers from a List
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers)
Flatten a Nested List
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flat_list = [num for sublist in matrix for num in sublist]
print(flat_list)
Dictionary Comprehensions
Create a Dictionary with Numbers as Keys and Their Squares as Values
diction = {i: i**2 for i in range(1, 6)}
print(diction)
Create a Dictionary from Two Lists
keys = ['a', 'b', 'c']
values = [1, 2, 3]
diction = {keys[i]: values[i] for i in range(len(keys))}
print(diction)
Filter a Dictionary to Keep Only Items Where the Value is Greater Than 2
original_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
filtered_dict = {k: v for k, v in original_dict.items() if v > 2}
print(filtered_dict)
Convert a Nested List into a Dictionary
nested_list = [['a', 1], ['b', 2], ['c', 3]]
d = {k: v for k, v in nested_list}
print(d)
Working with Tuples
Accessing Elements in a Tuple
my_tuple = (10, 20, 30, 40)
print(my_tuple[1])
Lambda Functions
Create a Lambda Function to Add Two Numbers
add = lambda x, y: x + y
print(add(5, 2))
Create a Lambda Function to Get the Square of a Number
square = lambda x: x**2
print(square(2))
Using map()
to Apply a Lambda Function to a List
numbers = [1, 2, 3, 4, 5]
squares = list(map(lambda x: x**2, numbers))
print(squares)
Using filter()
to Extract Even Numbers from a List
numbers = [1, 2, 3, 4, 5]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers)
Conclusion
List comprehensions, dictionary comprehensions, and lambda functions provide powerful tools for data processing in Python. These techniques help simplify code while maintaining efficiency. Understanding these fundamental concepts is essential for anyone working with data in Python.
We hope this article was helpful for you and you learned a lot about data analyst interview from it. If you have friends or family members who would find it helpful, please share it to them or on social media.
Join our social media for more.
Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your UnderstandingÂ
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.