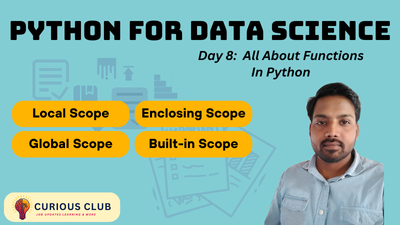
Welcome to Day 8 of Learning Python for Data Science. Today we will explore Functions in Python, which are fundamental building blocks for structuring code efficiently. Functions allow us to encapsulate reusable blocks of code, making programs more modular, readable, and easier to debug. In this article, we will dive deep into defining functions, using parameters and return values, and understanding real-world applications in data science.
Day 7 of Learning Python for Data Science – Tuples, Dictionaries, and Comprehensions
Functions in Python
Definition
A function is a block of code that performs a specific task and can be reused
Syntax
def function_name(parameters):
# Code block
return value
Key Concepts
- Parameters and Arguments
Parameters are the variables listed inside the parentheses in a function definition. They act as placeholders for values (called arguments) that are passed to the function when it is called.
- Return Statements
The return
statement is used in a function to send a value or multiple values back to the caller. Once a return
statement is executed, the function exits immediately, and the returned value can be stored in a variable or used directly.
- Scope of Variables
The scope of a variable in Python defines where the variable can be accessed or modified within a program. Python has four types of scopes based on the LEGB rule:
- Local Scope
- Enclosing Scope
- Global Scope
- Built-in Scope
1. Local Scope (Inside Function)
A variable declared inside a function is local and cannot be accessed outside the function.
def my_function():
x = 10 # Local variable
print(x)
my_function()
# print(x) # This would cause an error (NameError: name 'x' is not defined)
Rule: Local variables exist only inside the function where they are defined.
2. Enclosing Scope (Nested Functions)
When a function is inside another function, the inner function can access the variables of the outer function.
def outer():
y = "Python" # Enclosing variable
def inner():
print(y) # Inner function can access outer function's variable
inner()
outer()
The inner function can access the outer function’s variables, but the outer function cannot access the inner function’s variables.
3. Global Scope (Accessible Everywhere)
A variable declared outside any function is global and can be accessed inside functions.
z = 100 # Global variable
def my_function():
print(z) # Accessible inside function
my_function()
print(z) # Accessible outside function
Rule: Global variables can be accessed anywhere in the script.
Modifying Global Variables Inside a Function
To modify a global variable inside a function, use the global
keyword.
x = 5 # Global variable
def change_x():
global x
x = 10 # Modifying global variable
change_x()
print(x) # Output: 10
Warning: Modifying global variables inside functions is generally discouraged due to potential bugs.
4. Built-in Scope (Predefined by Python)
These are the variables and functions that Python provides by default, like print()
, len()
, etc.
print(len("Hello")) # 'len' is a built-in function
Rule: Built-in functions are always accessible unless overridden.
Summary
Scope Type | Where It’s Defined | Accessible In |
---|---|---|
Local (L) | Inside a function | Only inside that function |
Enclosing (E) | Outer function (nested) | Inside inner functions |
Global (G) | Outside any function | Anywhere in the script |
Built-in (B) | Predefined in Python | Always accessible |
Examples
Write a function to check if a number is odd or even.
def even_odd(n):
if n%2 == 0:
return 'even'
return 'Odd'
even_odd(8)
'Even'
Lambda function in Python
A lambda function in Python is a small, anonymous function that can have multiple arguments but only a single expression. It is defined using the lambda
keyword. These are widely used when a quick one time use function is required.
Syntax
lambda arguments: expression
- arguments → Inputs to the function
- expression → The single computation to return
Example: Basic Lambda Function
square = lambda x: x * x
print(square(5)) # Output: 25
This is equivalent to:
def square(x):
return x * x
Common Use Cases of Lambda Functions
1. Multiple Arguments in a Lambda Function
add = lambda x, y: x + y
print(add(3, 7)) # Output: 10
2. Using Lambda with map()
Applies a function to each element in a list.
numbers = [1, 2, 3, 4]
squared = list(map(lambda x: x**2, numbers))
print(squared) # Output: [1, 4, 9, 16]
3. Using Lambda with filter()
Filters elements based on a condition.
nums = [1, 2, 3, 4, 5, 6]
even_nums = list(filter(lambda x: x % 2 == 0, nums))
print(even_nums) # Output: [2, 4, 6]
4. Using Lambda with sorted()
Sorts a list based on a key function.
students = [("Alice", 25), ("Bob", 20), ("Charlie", 23)]
sorted_students = sorted(students, key=lambda x: x[1])
print(sorted_students)
# Output: [('Bob', 20), ('Charlie', 23), ('Alice', 25)]
5. Using Lambda in reduce()
Used for cumulative operations (requires functools.reduce
).
from functools import reduce
nums = [1, 2, 3, 4]
product = reduce(lambda x, y: x * y, nums)
print(product) # Output: 24
Lambda vs Regular Function
Feature | Lambda Function | Regular Function |
---|---|---|
Syntax | lambda x: x+2 | def func(x): return x+2 |
Function Name | Anonymous (or assigned to a variable) | Has a defined name |
Number of Expressions | Only one | Can have multiple |
Readability | Less readable for complex logic | More readable |
Introduction to Classes and Objects
Definition:
A class is a blueprint for creating objects, which are instances of a class.
Syntax:
class ClassName:
def __init__(self, parameters):
# Initialize attributes
def method_name(self):
# Code block
Key Concepts:
- Attributes (variables in a class)
- Methods (functions in a class)
- init Method (Constructor)
class intro:
def __init__(self,name,age):
self.name = name
self.age = age
def display(self):
print(f'My name is {self.name} and i am {self.age} years old')
test = intro('sneha',23)
test.display()
My name is sneha and i am 23 years old
class details:
# instance method
def __init__(self,name,age):
# instance variables
self.name = name
self.age = age
def display_info(self):
print(f'My name is {self.name} and i am {self.age} years old')
sneha_details = details('sneha',3)
sneha_details.display_info()
priya_details = details('priya',2)
priya_details.display_info()
My name is sneha and i am 3 years old
My name is priya and i am 2 years old
Basic OOP Concepts
- Encapsulation: Wrapping data and methods into a single unit (class).
- Inheritance: Deriving a new class from an existing class to reuse and extend functionality.
- Polymorphism: Using a single interface to represent different types.
Encapsulation is an OOP (Object-Oriented Programming) concept that restricts direct access to certain data or methods in an object. It helps prevent accidental modification of sensitive data.
class bank:
def __init__(self, name, balance):
self.name = name # Public variable
self.__balance = balance # Private variable (encapsulation)
def deposit(self, amount):
self.__balance += amount # Modify private variable inside class
def get_balance(self):
return self.__balance # Access private variable safely
amount = bank('sneha', 1000) # Creating an object
amount.deposit(200) # Depositing money
print(amount.get_balance()) # Output: 1200
print(amount.name) # Output: sneha
# print(amount.__balance) # This will raise an AttributeError
print(amount.__balance)
AttributeError: 'bank' object has no attribute '__balance'
Inheritance allows one class (child) to inherit attributes and methods from another class (parent).
class Vehicle:
def __init__(self,brand):
self.brand = brand
def show(self):
print(f'Brand name: {self.brand}')
class Car(Vehicle):
def __init__(self,brand,model):
super().__init__(brand)
self.model = model
def show_details(self):
print(f'Brand & Model : {self.brand}, {self.model}')
car1 = Car('Toyota','Camry')
car1.show()
car1.show_details()
Brand name: Toyota
Brand & Model : Toyota, Camry
In this example class car has inherited attributes and method from class vehicle.
Polymorphism allows the same method name to behave differently based on the object calling it.
class Animal:
def __init__(self,name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
print('Dog barks')
class Cat(Animal):
def speak(self):
print('Cat meows')
animals = [Dog('Buddy'),Cat('whiskers')]
for i in animals:
i.speak()
Dog barks
Cat meows
In the above code methos speak behaves differently when called for different classes.
Practice question
- What are the advantages of using functions in Python?
- How does the return statement work in Python functions?
- What is the difference between arguments and parameters in Python functions?
- Explain the concept of lambda functions with an example.
- What is the scope of a variable inside a function?
- How do you define a class in Python?
- What is the purpose of the init method in a class?
- How do you create an object from a class in Python?
- What is the difference between instance variables and class variables?
- What are the key principles of Object-Oriented Programming (OOP)?
- What is the purpose of defining functions in Python?
- How do lambda functions differ from regular functions?
- Write a function that takes a list of numbers and returns the sum.
- Modify the
factorial_iterative()
function to handle invalid inputs. - How does the
map()
function work, and when should you use it? - Write a lambda function to check if a number is prime.
- Create a function that filters out words shorter than 4 letters from a list.
- Implement a recursive function to compute the Fibonacci sequence.
- What are the advantages of using higher-order functions in data science?
- Compare
map()
andfilter()
, providing an example for each.
We hope this article was helpful for you and you learned a lot about data analyst interview from it. If you have friends or family members who would find it helpful, please share it to them or on social media.
Join our social media for more.
Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your UnderstandingÂ
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.