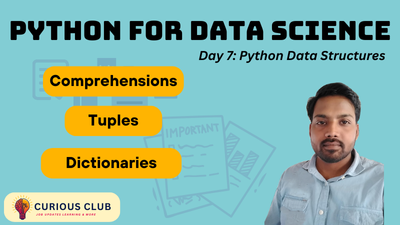
Introduction
Welcome to Day 7 of Learning Python for Data Science. Today we will see that Tuples, dictionaries, and comprehensions are essential Python data structures that enable efficient data handling and manipulation. They are widely used in data science applications for storing and processing structured data. In this article, we will explore these structures in-depth, covering their methods, operations, and real-world applications.
Tuples in Python
What is a Tuple?
A tuple is an immutable, ordered sequence of elements that allows duplicate values. It is similar to a list but cannot be modified after creation, making it useful for fixed data collections.
Creating Tuples
# Creating tuples with different types of elements
tuple1 = (1, 2, 3)
tuple2 = ('apple', 'banana', 'cherry')
tuple3 = (1, 'hello', 3.14)
Output:
(1, 2, 3)
('apple', 'banana', 'cherry')
(1, 'hello', 3.14)
Accessing Elements
# Indexing and Slicing
tuple1[0] # First element
tuple1[-1] # Last element
tuple1[1:3] # Slicing
Output:
1
3
(2, 3)
Tuple Operations
Concatenation:
tuple1 = (1, 2, 3)
tuple2 = ('apple', 'banana', 'cherry')
tuple1 + tuple2
Output:
(1, 2, 3, 'apple', 'banana', 'cherry')
Repetition:
tuple1 = (1, 2, 3)
tuple1 * 3
Output:
(1, 2, 3, 1, 2, 3, 1, 2, 3)
Length:
len(tuple1)
Output:
3
Finding occurrences:
tuple1.count(2)
Output:
1
Finding index:
tuple1.index(3)
Output:
2
Checking membership:
3 in tuple1
Output:
True
Sorting a tuple:
sorted(tuple1) # returns a list
Output:
[1, 2, 3]
Reversing a tuple:
tuple1[::-1]
Output:
(3, 2, 1)
Finding maximum and minimum values:
max(tuple1)
min(tuple1) # (only for numerical tuples)
Output:
3
1
Converting tuple to string:
''.join(tuple2) # (only for string elements)
Output:
'applebananacherry'
Tuple Unpacking
# Unpacking a tuple
a, b, c = tuple1
Output:
1 2 3
marks = ([10,40],50,10,20,30,[12,13,14])
a,b,c,*d= marks
print(a,b,c,d)
Output:
10 40 50 [10, 20, 30, [12, 13, 14]] # d stored all the remaining values
Iterating Through Tuples
for item in tuple1:
print(item)
Output:
1
2
3
Converting Between Tuples and Lists
list1 = list(tuple1)
tuple4 = tuple(list1)
Output:
[1, 2, 3] <class 'list'>
(1, 2, 3) <class 'tuple'>
Dictionaries in Python
What is a Dictionary?
A dictionary is an unordered collection of key-value pairs. It allows fast lookups and modifications based on unique keys.
Creating Dictionaries
dict1 = {'name': 'Alice', 'age': 25, 'city': 'New York'}
Output:
{'name': 'Alice', 'age': 25, 'city': 'New York'}
Accessing Values
name = dict1['name'] # Direct access
age = dict1.get('age') # Using get method
Output:
'Alice'
25
Adding and Updating Values
dict1['age'] = 26
dict1['country'] = 'USA'
Output:
{'name': 'Alice', 'age': 26, 'city': 'New York', 'country': 'USA'}
Dictionary Methods
Removing an item:
dict1.pop('age')
Output:
26
Removing the last item:
dict1.popitem()
Output:
('country', 'USA')
Merging dictionaries:
dict1.update(dict2)
Output:
{'name': 'Alice', 'city': 'New York', 'age': 25}
Clearing dictionary:
dict1.clear()
Output:
{}
Getting keys, values, and items:
dict1.keys()
dict1.values()
dict1.items()
Output:
Keys: dict_keys(['name', 'age', 'city'])
Values: dict_values(['Alice', 25, 'New York'])
Items: dict_items([('name', 'Alice'), ('age', 25), ('city', 'New York')])
Checking key existence:
'name' in dict1
Output:
True
Copying a dictionary:
dict_copy = dict1.copy()
Output:
{'name': 'Alice', 'age': 25, 'city': 'New York'} # creates a copy
Setting a default value:
dict1.setdefault('gender', 'Female')
Output:
'Female'
Updating multiple values:
dict1.update({'age': 30, 'city': 'Los Angeles'})
Output:
{'name': 'Alice', 'age': 30, 'city': 'Los Angeles', 'gender': 'Female'}
Creating a dictionary from keys:
dict.fromkeys(['name', 'age'], 'Unknown')
Output:
{'name': 'Unknown', 'age': 'Unknown'}
Iterating Through Dictionaries
for key, value in dict1.items():
print(f'{key}: {value}')
Output:
name: Alice
age: 30
city: Los Angeles
gender: Female
Nested Dictionaries
dict2 = {'student1': {'name': 'John', 'marks': 85}, 'student2': {'name': 'Jane', 'marks': 90}}
Output:
{'student1': {'name': 'John', 'marks': 85}, 'student2': {'name': 'Jane', 'marks': 90}}
Converting Dictionary to List and JSON
list_keys = list(dict1.keys())
list_values = list(dict1.values())
import json
dict_json = json.dumps(dict1)
Output:
'{"name": "Alice", "age": 30, "city": "Los Angeles", "gender": "Female"}'
List, Set, and Dictionary Comprehensions
List Comprehensions
squares = [x ** 2 for x in range(10)]
Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
even_numbers = [x for x in range(10) if x % 2 == 0]
Output: [0, 2, 4, 6, 8]
Set Comprehensions
unique_vowels = {char for char in 'hello world' if char in 'aeiou'}
Output: {'e', 'o'}
Dictionary Comprehensions
squares_dict = {x: x**2 for x in range(5)}
Output: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
filtered_dict = {k: v for k, v in dict1.items() if isinstance(v, int)}
Output: {'age': 30}
Practice Questions
Tuples
- Create a tuple with numbers and find the maximum and minimum values.
- Write a program to count occurrences of an element in a tuple.
- Convert a tuple of strings to a single concatenated string.
- Check if an element exists in a tuple.
- Swap two tuples without using a temporary variable.
- Reverse a tuple.
- Write a program to find common elements between two tuples.
- Convert a tuple of numbers into a tuple of their squares.
- Merge two tuples and remove duplicates.
- Write a function to return the first and last element of a tuple.
Dictionaries
- Create a dictionary from two lists (keys and values).
- Write a function that returns keys with the highest values in a dictionary.
- Merge two dictionaries and sort by key.
- Count occurrences of characters in a string using a dictionary.
- Find the sum of all values in a dictionary.
- Remove a key from a dictionary safely.
- Sort a dictionary by its values in ascending order.
- Write a program to invert keys and values in a dictionary.
- Create a dictionary with keys as numbers and values as their cubes.
- Convert a dictionary to a list of tuples.
Comprehensions
- Generate a list of squares of even numbers from 1 to 20.
- Create a set of unique characters from a given string using comprehension.
- Write a dictionary comprehension to filter out keys that have even values.
- Generate a list of words that have more than 3 letters from a sentence.
- Create a dictionary where keys are numbers from 1 to 10, and values are their factorials.
- Extract unique words from a paragraph using set comprehension.
- Convert a list of tuples into a dictionary using comprehension.
- Generate a list of numbers that are multiples of both 3 and 5 from 1 to 100.
- Create a dictionary with student names as keys and their grades as values, filtering only students with grades above 80.
- Generate a set of squares of odd numbers from 1 to 30.
Click here for solutions – Python Practice Questions & Solutions
This article provides a comprehensive guide to tuples, dictionaries, and comprehensions, along with hands-on practice and exercises to reinforce learning. Happy coding!
Join our social media for more.
Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your UnderstandingÂ
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.