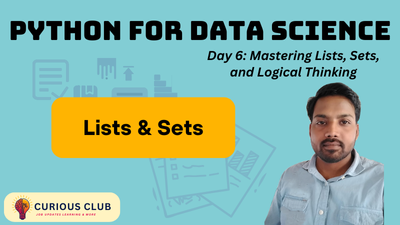
Introduction to Data Structures
Data structures are the backbone of programming, enabling efficient organization and manipulation of data. Python provides several built-in data structures, such as:
- Lists – Ordered collections of elements that allow duplicates.
- Tuples – Immutable sequences for fixed data storage.
- Sets – Unordered collections containing only unique elements.
- Dictionaries – Mappings of key-value pairs for fast lookups.
In practical applications:
- A list can store a class’s student names.
- A set ensures roll numbers remain unique.
- Ordered collection means list will store the elements in order of insertion.
- Unordered collection means set does not maintain the order of insertion and each time you try accessing the collection the order will be different.
Lists
Definition and Properties
A list is a mutable, ordered collection that can store multiple data types.
Creating a list
students = ["Alice", "Bob", "Charlie"]
print(students) # Output: ['Alice', 'Bob', 'Charlie']
Common List Operations
Python offers several methods for manipulating lists:
Adding elements
students.append("David") # Appends 'David' at the end
students.insert(1, "Eve") # Inserts 'Eve' at index 1
Removing elements
students.remove("Bob") # Removes 'Bob'
students.pop() # Removes the last element
Slicing lists
print(students[1:3]) # Outputs elements from index 1 to 2
Modifying elements
name = ['Vishal', 'Deepanjan', 'Satyam']
name[0] = 'Jaiswal'
print(name)
['Jaiswal', 'Deepanjan', 'Satyam']
Common List Methods:
append()
– Adds an element at the end of the list.
students = ["Alice", "Bob"]
students.append("Charlie")
print(students) # Output: ['Alice', 'Bob', 'Charlie']
insert()
– Inserts an element at a specific index.
students.insert(1, "David")
print(students) # Output: ['Alice', 'David', 'Bob', 'Charlie']
remove()
– Removes a specific element from the list.
students.remove("Bob")
print(students) # Output: ['Alice', 'David', 'Charlie']
pop()
– Removes and returns the last element.
last_student = students.pop()
print(last_student) # Output: 'Charlie'
print(students) # Output: ['Alice', 'David']
Slicing (list[start:end]
) – Extracts a portion of the list.
numbers = [10, 20, 30, 40, 50]
print(numbers[1:4]) # Output: [20, 30, 40]
len()
– Returns the number of elements in the list.
print(len(numbers)) # Output: 5
sort()
– Sorts the list in ascending order.
numbers.sort()
print(numbers) # Output: [10, 20, 30, 40, 50]
reverse()
– Reverses the elements of the list.
numbers.reverse()
print(numbers) # Output: [50, 40, 30, 20, 10]
index()
– Returns the index of the first occurrence of an element.
print(numbers.index(30)) # Output: 2
Sets
Definition and Significance
A set is an unordered, mutable collection that stores only unique elements.
Creating a set
unique_numbers = {1, 2, 3, 3, 4, 5}
print(unique_numbers) # Output: {1, 2, 3, 4, 5}
Set Operations
Python supports various set operations for mathematical and logical manipulations:
Common Set Methods:
union()
– Combines two sets, removing duplicates.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1.union(set2)) # Output: {1, 2, 3, 4, 5}
intersection()
– Returns common elements between two sets.
print(set1.intersection(set2)) # Output: {3}
difference()
– Returns elements present in the first set but not in the second.
print(set1.difference(set2)) # Output: {1, 2}
len()
– Returns the number of elements in a set.
print(len(set1)) # Output: 3
add()
– Adds a single element to the set.
set1.add(6)
print(set1) # Output: {1, 2, 3, 6}
update()
– Adds multiple elements to the set.
set1.update([7, 8, 9])
print(set1) # Output: {1, 2, 3, 6, 7, 8, 9}
discard()
– Removes an element from the set without error if it doesn’t exist.
set1.discard(2)
print(set1) # Output: {1, 3, 6, 7, 8, 9}
remove()
– Removes an element but raises an error if it doesn’t exist.
set1.remove(3)
print(set1) # Output: {1, 6, 7, 8, 9}
pop()
– Removes and returns an arbitrary element from the set.
print(set1.pop()) # Output: (random element from the set)
clear()
– Removes all elements from the set.
set1.clear()
print(set1) # Output: set()
issubset()
– Checks if all elements of a set exist in another set.
set1 = {1, 2, 3}
set2 = {1, 2, 3, 4, 5}
print(set1.issubset(set2)) # Output: True
issuperset()
– Checks if a set contains all elements of another set.
print(set2.issuperset(set1)) # Output: True
isdisjoint()
– Returns True if two sets have no common elements.
set3 = {6, 7, 8}
print(set1.isdisjoint(set3)) # Output: True
copy()
– Returns a shallow copy of the set.
set_copy = set1.copy()
print(set_copy) # Output: {1, 2, 3}
Practice Questions
- Create a list of five student names and print it.
- Add a new student name to the end of the list using the appropriate method.
- Insert a student name at the second position in the list.
- Remove a specific student name from the list.
- Remove and return the last element of the list.
- Retrieve a sublist containing elements from index 2 to 4 using slicing.
- Find the index of a specific student name in the list.
- Sort a list of integers in ascending order.
- Reverse the order of elements in a list.
- Count the number of times a specific element appears in a list.
- Find the length of a given list.
- Create a set containing five unique numbers and print it.
- Add a new element to the set.
- Try adding a duplicate element to the set and observe the output.
- Remove an element from the set using the appropriate method.
- Perform a union operation between two sets and print the result.
- Find the common elements between two sets using the intersection method.
- Find the elements that exist in the first set but not in the second set using the difference method.
- Check if one set is a subset of another set.
- Check if two sets have no common elements using the appropriate method.
- Write a Python program to count the number of occurrences of each element in a given list.
- Create a set of numbers from 1 to 10. Find all the numbers that are divisible by 3 using a set operation.
- Given two lists of integers, write a program to find the elements that are common in both lists using a set.
- Write a program to remove duplicate words from a given string and display the unique words in alphabetical order.
- Create a list of mixed data types (e.g., numbers and strings). Write a program to separate the strings and numbers into two different lists.
- Write a function that returns the second largest number from a list of integers. Example:
[10, 20, 4, 45, 99]
→ Output:45
- Given a list and an integer
k
, shift elements to the right byk
positions. Example:([1, 2, 3, 4, 5], k=2)
→ Output:[4, 5, 1, 2, 3]
- Given a list, remove duplicate elements while maintaining the order. Example:
[1, 2, 2, 3, 4, 4, 5]
→ Output:[1, 2, 3, 4, 5]
- Given a list and a target sum, find all unique pairs whose sum equals the target. Example:
([1, 2, 3, 4, 5, 6], target=7)
→ Output:[(1, 6), (2, 5), (3, 4)]
- Implement a function that finds the index of a given element in a list manually. Example:
([10, 20, 30, 40], target=30)
→ Output:2
- Write a function that returns elements that exist in either of the two sets but not both. Example:
{1, 2, 3, 4}
and{3, 4, 5, 6}
→ Output:{1, 2, 5, 6}
- Given two sets, determine if one set is a subset of the other. Example:
{1, 2}
is a subset of{1, 2, 3, 4}
→ Output:True
- Given multiple sets, find the total count of unique elements across all sets. Example:
[{1, 2, 3}, {3, 4, 5}, {5, 6, 7}]
→ Output:7
- Given three sets, find the elements that are common in all three. Example:
{1, 2, 3}
,{2, 3, 4}
,{3, 4, 5}
→ Output:{3}
- Remove all elements of
set2
fromset1
without using a loop. Example:{1, 2, 3, 4, 5}
minus{3, 4}
→ Output:{1, 2, 5}
- Given a list of integers, find the length of the longest consecutive elements sequence. Example: [100, 4, 200, 1, 3, 2] → Output: 4 ([1, 2, 3, 4] is the longest sequence)
- Rearrange a sorted list so that the first element is the smallest, the second is the largest, the third is the second smallest, and so on. Example: [1, 2, 3, 4, 5, 6, 7] → Output: [1, 7, 2, 6, 3, 5, 4]
- An element is a majority if it appears more than n/2 times in a list of size n. Find it in O(n) time. Example: [3, 3, 4, 2, 4, 4, 2, 4, 4] → Output: 4
- Given two sets of numbers, find all pairs (a, b) where a is from set1, b is from set2, and (a + b) is even. Example: {1, 2, 3} and {4, 5, 6} → Output: {(2, 4), (2, 6), (1, 5), (3, 5)}
Click here for solutions – Python Practice Questions & Solutions
Conclusion
On Day 6 of Python Learning, we explored:
- The characteristics and manipulation of lists.
- The properties and advantages of sets.
- Problem-solving strategies involving lists and sets.
Mastering these concepts will significantly enhance your data-handling skills in Python, paving the way for advanced topics in data science and algorithm design.
We hope this article was helpful for you and you learned a lot about data analyst interview from it. If you have friends or family members who would find it helpful, please share it to them or on social media.
Join our social media for more.
Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your UnderstandingÂ
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.