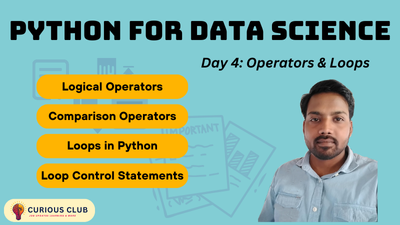
Day 4 of Learning Python for Data Science: Master essential concepts like data structures, loops, and functions. Explore hands-on coding examples and real-world applications to boost your Python skills for data analysis and machine learning. read more
Today, we will explore essential programming concepts that will help you write efficient and logical code. The focus areas for today include:
✅ Comparison Operators (To compare values)
✅ Logical Operators (To combine conditions)
✅ Loops in Python (To execute code multiple times)
✅ Loop Control Statements (To manage loops effectively)
Comparison Operators in Python
Comparison operators allow us to compare two values and return a Boolean (True
or False
).
Operator | Description | Example | Output |
---|---|---|---|
== | Equal to | 5 == 5 | True |
!= | Not equal to | 5 != 3 | True |
> | Greater than | 10 > 3 | True |
< | Less than | 2 < 5 | True |
>= | Greater than or equal to | 7 >= 7 | True |
<= | Less than or equal to | 3 <= 5 | True |
Example
a = 10
b = 5
print(a == b) # False
print(a != b) # True
print(a > b) # True
print(a < b) # False
print(a >= 10) # True
print(b <= 5) # True
Output
False
True
True
False
True
True
Logical Operators in Python
Logical operators are used to combine multiple conditions.
Operator | Description | Example | Output |
---|---|---|---|
and | Returns True if both conditions are True | (5 > 3) and (10 > 5) | True |
or | Returns True if at least one condition is True | (5 > 3) or (10 < 5) | True |
not | Reverses the Boolean result | not(5 > 3) | False |
Example
x = 7
y = 10
print(x > 5 and y < 15) # True (Both conditions are True)
print(x > 10 or y < 15) # True (At least one condition is True)
print(not (x > 5)) # False (Reverses True to False)
Output
True
True
False
Loops in Python
Loops allow us to repeat a block of code multiple times without writing redundant code.
For Loop
A for
loop is used when you know how many times you want to repeat the code.
for i in range(1, 6): # Loops from 1 to 5
print("Iteration:", i)
Output
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Iteration: 5
While Loop
A while
loop runs until a condition becomes False.
count = 1
while count <= 3:
print("Count:", count)
count += 1
Output
Count: 1
Count: 2
Count: 3
Loop Control Statements
These statements modify loop execution.
break
(Exit the loop early)
for i in range(1, 6):
if i == 3:
break # Stops the loop when i equals 3
print(i)
Output
1
2
continue
(Skip the current iteration)
for i in range(1, 6):
if i == 3:
continue # Skips when i is 3
print(i)
Output
1
2
4
5
pass
(Placeholder statement)
for i in range(3):
pass # Does nothing, avoids syntax errors
print("Loop executed successfully")
Output
Loop executed successfully
Practice Questions
Test your understanding with these 20 practice questions on comparison operators, logical operators, loops, and loop control statements.
What will be the output of this code?
print(10 == 10)
Write a Python program that checks whether a number is positive, negative, or zero.
What will be the output?
print(5 > 3 and 10 > 5)
Write a program to check if a number is divisible by both 2 and 5 using logical operators.
What will be the output of this while
loop?
x = 1
while x < 5:
print(x)
x += 1
Modify the code below to use a for
loop instead of while
:
x = 1
while x <= 5:
print(x)
x += 1
What happens if the break
statement is removed from the following loop?
for i in range(1, 6):
if i == 4:
break
print(i)
Write a program that prints all even numbers from 1 to 20 using a loop.
What will be the output of the following?
for i in range(1, 6):
if i == 3:
continue
print(i)
Write a Python program that skips all numbers divisible by 3 in a loop from 1 to 10 using continue
.
Write a for
loop that prints numbers from 1 to 10 but stops when it reaches 7.
What will be the output?
x = 5
y = 10
print(x > 2 and y < 15)
Write a program that calculates the sum of numbers from 1 to 100 using a for
loop.
Modify the following loop to stop execution when x
is greater than 50.
x = 1
while x < 100:
print(x)
x += 5
Write a program that counts how many even numbers exist between 1 and 50.
What will be the output of the following?
x = 10
if x > 5:
print("Greater")
if x > 8:
print("Higher")
if x > 15:
print("Highest")
Modify the following loop to use pass
when x == 5
.
for x in range(10):
print(x)
Print even numbers from 1 to 20 using a for loop.
Print the sum of numbers from 1 to 50 using a while loop.
Print the reverse of a given string using a loop.
Use a loop to find the factorial of a number.
Generate the Fibonacci series up to 10 terms using a while loop
Use a for loop to check if a number is prime.
Today, we explored comparison operators, logical operators, loops, and loop control statements in Python. These are fundamental concepts for writing logical and efficient code.
👉 Next Steps:
✅ Try solving all practice questions – LINK FOR SOLUTIONS
✅ Check the next article for solutions!
🔗 Stay tuned for Day 5 of Learning Python!
We hope this article was helpful for you and you learned a lot about data analyst interview from it. If you have friends or family members who would find it helpful, please share it to them or on social media.
Join our social media for more.
Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your UnderstandingÂ
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.