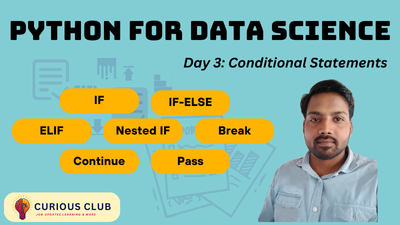
Understanding Python’s conditional statements is essential for controlling the flow of a program. Today, we will explore if-else
conditions, nested conditions, and special control statements like break
, continue
, and pass
.
Conditional Statements
If Statement
Definition: The if
statement is used to execute a block of code only if a specified condition is true.
Example:
x = 10
if x > 5:
print("x is greater than 5")
Output:
x is greater than 5
If-Else Statement
Definition: The if-else
statement allows us to execute one block of code when a condition is true and another when it is false.
Example:
num = 7
if num % 2 == 0:
print("Even")
else:
print("Odd")
Output:
Odd
Elif Statement
Definition: The elif
statement allows checking multiple conditions sequentially.
Example:
score = 85
if score >= 90:
print("Excellent")
elif score >= 75:
print("Good")
else:
print("Needs Improvement")
Output:
Good
Nested If Statements
Definition: A nested if
statement is an if
statement inside another if
statement, allowing multiple layers of conditions.
Example:
x = 15
if x > 10:
if x < 20:
print("Between 10 and 20")
Output:
Between 10 and 20
Break Statement
Definition: The break
statement exits a loop or control structure prematurely when a specified condition is met.
Example:
for i in range(10):
if i == 5:
break
print(i)
Output:
0
1
2
3
4
Continue Statement
Definition: The continue
statement skips the current iteration and moves to the next one.
Example:
for i in range(5):
if i == 2:
continue
print(i)
Output:
0
1
3
4
Pass Statement
Definition: The pass
statement is a placeholder that allows defining an empty code block without causing an error.
Example:
def my_function():
pass # Placeholder for future code
(No output as pass
does nothing.)
Practice Questions
What will be the output of the following code?
x = 10
if x > 5:
print("Greater")
else:
print("Smaller")
Write a Python program using an if-else statement to check if a number is positive, negative, or zero.
What will be the output of the following code?
num = 7
if num % 2 == 0:
print("Even")
else:
print("Odd")
What will be the output of the following nested if condition?
x = 15
if x > 10:
if x < 20:
print("Between 10 and 20")
Write a Python program that uses nested if statements to check whether a given number is positive, even, and greater than 10.
What is the output of the following program?
pythonCopyEditx = 5
y = 10
if x > 2:
if y > 5:
print("Valid")
Write a Python program that asks for a user’s age and prints whether they are a child (0-12), teenager (13-19), adult (20-59), or senior (60+).
What will be the output of the following code?
x = 50
if x >= 50:
if x == 50:
print("Exactly 50")
else:
print("Greater than 50")
else:
print("Less than 50")
Write a Python program using the pass
statement inside an empty if
condition.
What will the following program print?
x = 20
y = 30
if x < y:
if x + 10 == y:
print("Match")
Write a Python program that prints whether a number is positive or negative using an if-else statement.
Modify the code below to use an elif condition to check whether a number is positive, negative, or zero.
num = int(input("Enter a number: "))
if num > 0:
print("Positive")
else:
print("Negative or Zero")
What is the output of this code snippet?
x = 10
if x > 5:
print("High")
if x > 8:
print("Higher")
if x > 15:
print("Highest")
Write a program that takes a number as input and prints whether it is divisible by both 2 and 3 using a nested if statement.
Write a Python program that checks if a number is a multiple of both 4 and 6 using a nested if
statement.
Modify the program below to check whether a number is even and greater than 10.
num = int(input("Enter a number: "))
if num % 2 == 0:
print("Even")
Write a Python program using if-elif-else conditions to categorize a number into small (1-10), medium (11-50), or large (above 50).
What is the output of this nested condition?
x = 3
y = 9
if x < 5:
if y > 8:
print("Success")
Modify the program below to check if a number is between 10 and 50 using a conditional statement.
num = int(input("Enter a number: "))
if num > 10:
print("Valid")
Write a Python program that checks if a number is prime using conditional statements.
The answer for these is shared here: click here for answers
Conclusion
This lesson covers essential control flow structures in Python, such as conditional statements and control keywords. Understanding these concepts is crucial for writing efficient and logical Python programs. In the next session, we will explore loops and how they help automate repetitive tasks in Python.
We hope this article was helpful for you and you learned a lot about data analyst interview from it. If you have friends or family members who would find it helpful, please share it to them or on social media.
Join our social media for more.
Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science Python for Data Science
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your UnderstandingÂ
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.