In this article, we will dive deep to understand hstack and vstack, exploring their usage, advantages, and practical implementations.
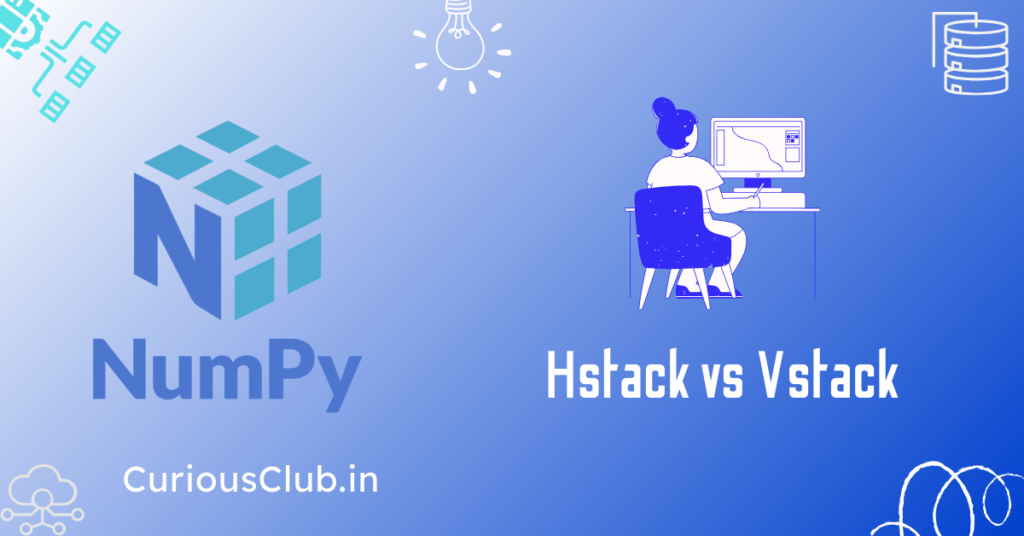
Understanding hstack and vstack
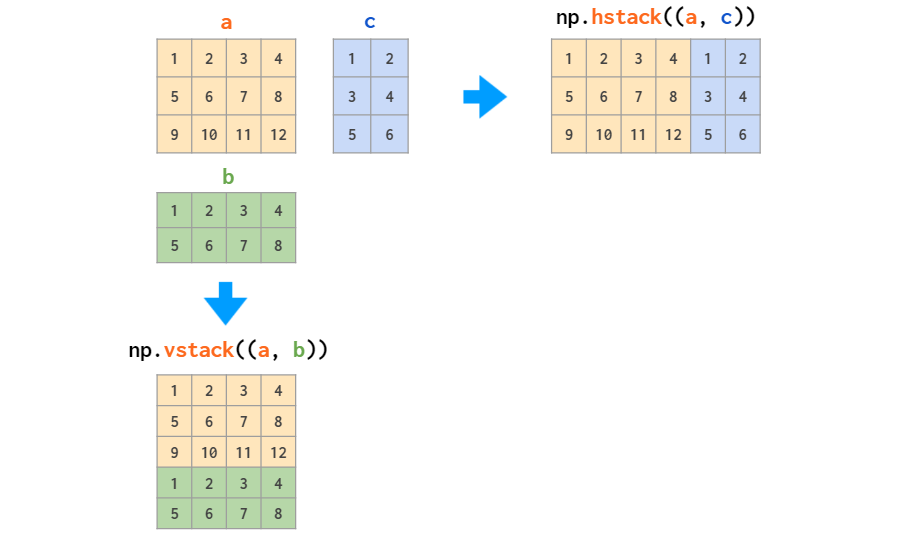
hstack (Horizontal Stack):
hstack()
horizontally concatenates arrays along their columns.- It extends arrays along the second axis (axis=1), resulting in a larger number of columns.
- Primarily used for merging arrays with identical row counts.
vstack (Vertical Stack):
vstack()
vertically concatenates arrays along their rows.- It extends arrays along the first axis (axis=0), leading to an increased number of rows.
- Ideal for appending data vertically, particularly when arrays share the same column counts.
Usage and Syntax
hstack:
- Syntax:
numpy.hstack(tup)
tup
: Sequence of arrays to be horizontally stacked.
vstack:
- Syntax:
numpy.vstack(tup)
tup
: Sequence of arrays to be vertically stacked.
Benefits and Applications
Data Integration:
hstack()
facilitates the merging of datasets with complementary attributes but identical row structures.vstack()
enables the seamless addition of new observations to existing datasets without compromising column consistency.
Matrix Operations:
- Utilize
hstack()
for horizontally concatenating matrices, thus facilitating the construction of larger matrices. - Leverage
vstack()
to vertically stack matrices, facilitating column-wise aggregations and extensions.
Practical Examples
Horizontal Stack:
import numpy as np
arr1 = np.array([[1, 2], [3, 4]])
arr2 = np.array([[5, 6], [7, 8]])
stacked_arr = np.hstack((arr1, arr2))
print(stacked_arr)
[[1 2 5 6]
[3 4 7 8]]
Vertical Stack:
import numpy as np
arr1 = np.array([[1, 2], [3, 4]])
arr2 = np.array([[5, 6], [7, 8]])
stacked_arr = np.vstack((arr1, arr2))
print(stacked_arr)
[[1 2 5 6]
[3 4 7 8]]
Conclusion
Mastery of hstack()
and vstack()
empowers efficient array manipulation in NumPy, facilitating diverse operations such as data merging and matrix construction. By integrating these functions into your workflows, you unlock enhanced flexibility and productivity in array-based computations. Experimentation with hstack()
and vstack()
not only streamlines data manipulation tasks but also broadens your capabilities in numerical computing.
We hope that you liked our information, if you liked our information, then you must share it with your friends, family and group. So that they can also get this information.
Also Read:
- Demystifying NumPy Copy and NumPy View – CuriousClub
- Pandas Series: Unleash the Power of Data
- NumPy Delete
- NumPy Insert
- NumPy Append
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.
1 thought on “Mastering hstack and vstack in NumPy”