NumPy, the cornerstone of numerical computing in Python, introduces two key concepts: “numpy copy” and “numpy view.” Let’s unravel these concepts concisely, exploring their differences, applications, and impact on array operations.
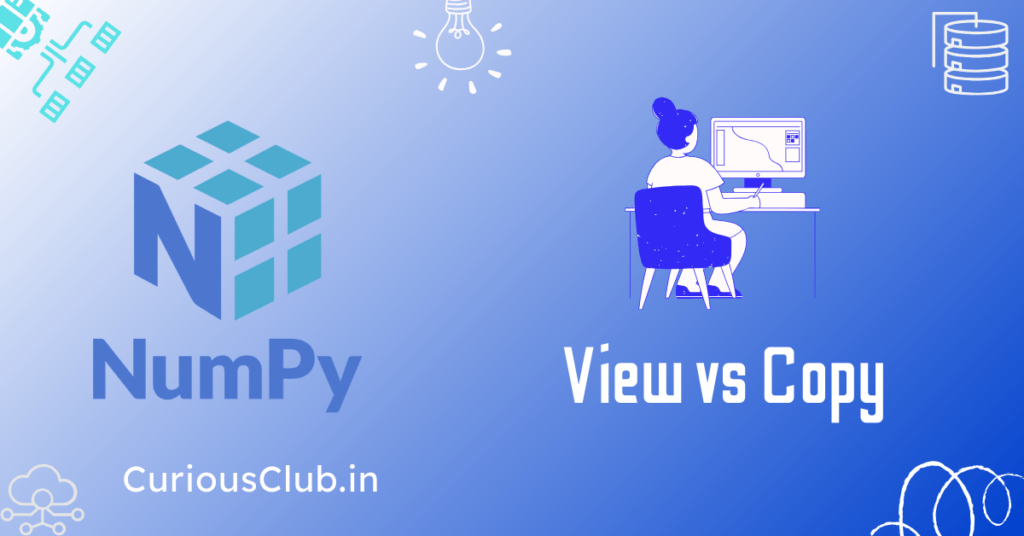
Copy vs. View: Unveiling the Contrast
NumPy Copy:
- Represents an independent array with its own data.
- Modifications to a copy don’t affect the original array and vice versa.
- Incurs memory overhead due to separate data storage.
NumPy View:
- Offers an alternative perspective of the same data.
- Shares data storage with the original array but may exhibit different shapes or strides.
- Modifications to a view reflect in the original array and vice versa.
- Memory-efficient as it avoids duplicating data.
Creating Copies and Views
Copy Creation:
- Employ
copy()
method ornp.copy()
function for deep copying.
# Create an array
original_arr = np.array([1, 2, 3, 4, 5])
# Create a copy using the copy() method
copy_using_method = original_arr.copy()
# Modify the original array
original_arr[0] = 100
# Print the original array and the copy to observe changes
print("Original Array:", original_arr)
print("Copy using copy() method:", copy_using_method)
Original Array: [100 2 3 4 5]
Copy using copy() method: [1 2 3 4 5]
In this example:
- We create an original NumPy array
original_arr
. - We create a copy of the original array using the
copy()
method. - We then modify the first element of the original array.
- Finally, we print both the original array and the copy to observe that modifications to the original array do not affect the copy, confirming that
copy_using_method
is indeed a deep copy.
Slicing, reshaping, transposing, and certain broadcasting operations yield views.
- Slicing:
- When you slice a NumPy array, you create a view of the original array. This view refers to the same data in memory but presents it in a different shape or with different strides.
- Slicing operations allow you to extract subsets of an array without copying the data, making them memory-efficient.
- Reshaping:
- The
reshape()
method in NumPy allows you to change the shape of an array without changing its data. - Reshaping an array creates a view of the original array with a new shape. The data remains the same, but the new view may have different dimensions.
- The
- Transposing:
- The
transpose()
method in NumPy allows you to interchange the axes of an array. - Transposing an array creates a view of the original array with the axes reordered. Again, the data remains the same, but the view presents it differently.
- The
- Broadcasting:
- Broadcasting in NumPy refers to a set of rules for performing element-wise operations on arrays with different shapes.
- In broadcasting, arrays are automatically reshaped or duplicated to match each other’s shapes before the operation is applied.
- Broadcasting operations create views of the original arrays, allowing for efficient computation without copying data.
View Creation:
- Use
view()
method for explicit view creation.
# Create an array
original_arr = np.array([[1, 2, 3],
[4, 5, 6]])
# Create a view of the original array with a different shape
view_arr = original_arr.view()
# Modify the original array
original_arr[0, 0] = 100
# Print both the original array and the view to observe changes
print(original_arr) : [[100 2 3]
[ 4 5 6]]
print(view_arr) : [[100 2 3]
[ 4 5 6]]
Effects on Array Operations
- Performance Impact:
- Views outshine copies in performance, especially with substantial datasets.
- Copies necessitate extra memory and computational resources.
- Memory Management:
- Copies inflate memory usage, particularly with large datasets.
- Views conserve memory by sharing data with the original array.
In Conclusion
Understanding copy and view in NumPy is pivotal for efficient data handling and memory utilization. Mastery of these concepts empowers optimization of code for performance and memory efficiency, facilitating seamless data analysis. Experimentation with copies and views in NumPy workflows enhances productivity and computational effectiveness.
We hope that you liked our information, if you liked our information, then you must share it with your friends, family and group. So that they can also get this information.
Also Read:
- Introduction to NumPy | NumPy Array | NumPy – CuriousClub
- Pandas Series: Unleash the Power of Data
- NumPy Delete
- NumPy Insert
- NumPy Append
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.