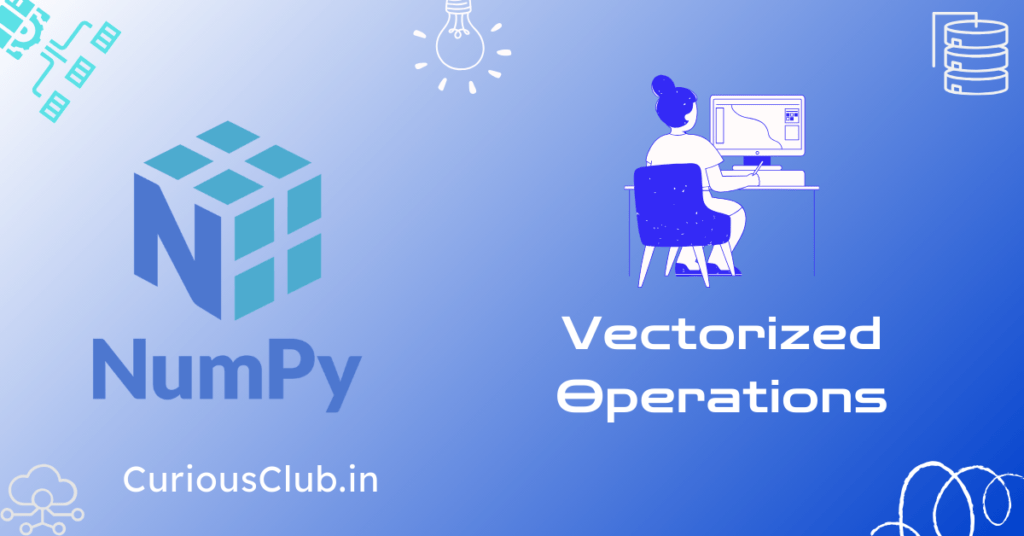
What are Vectorized Operations?
Imagine you have a list of numbers and want to calculate the square of each element. Traditionally, you might use a for
loop to iterate through the list, performing the calculation on each item individually. This method works, but for large datasets, it can be slow and inefficient.
Vectorized operations offer a more efficient alternative. Instead of looping through each element, vectorized operations leverage built-in functions and mathematical operators in libraries like NumPy to perform calculations on entire arrays (sequences of data) simultaneously. Think of it as processing multiple values in a single step, achieving significant speed improvements.
Benefits of Vectorized Operations:
- Blazing Speed:Â Vectorized operations are optimized for handling large datasets. By leveraging compiled code (often written in C), they outperform loops by a significant margin, especially when dealing with thousands or millions of data points.
- Concise and Readable Code:Â Vectorized code is often shorter and easier to understand compared to loop-based approaches. It eliminates repetitive loops and focuses on the core logic of the operation, making your code more maintainable and less prone to errors.
- Maintainability Advantage:Â Concise vectorized code is easier to maintain and debug. You’ll spend less time wrestling with complex loops and more time focusing on the bigger picture of your project.
Vectorized Operations in Action:
Let’s see how vectorized operations work in practice. Here’s an example of calculating the square of each element in a list:
Non-vectorized approach (using a loop):
numbers = [1, 2, 3, 4, 5]
squared_numbers = []
for num in numbers:
squared_numbers.append(num * num)
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
Vectorized approach (using NumPy):
import numpy as np
numbers = np.array([1, 2, 3, 4, 5])
squared_numbers = numbers * numbers
print(squared_numbers) # Output: [1 4 9 16 25]
In this example, we import the NumPy library (import numpy as np
) to work with arrays. The numbers
list is converted into a NumPy array. Then, the *
operator performs element-wise multiplication, calculating the square of each element in a single line. This demonstrates the power and simplicity of vectorized operations.
Beyond NumPy:
Vectorized operations extend beyond NumPy. Libraries like Pandas (data manipulation) and SciPy (scientific computing) also offer optimized functions for specific data types and tasks. By embracing vectorization across these libraries, you’ll unlock a whole new level of efficiency in your Python data science workflow.
Ready to Supercharge Your Python Skills?
Vectorized operations are an essential tool for anyone working with numerical data in Python. By incorporating them into your coding practices, you’ll experience significant speed improvements, write cleaner code, and streamline your data analysis tasks.
Also Read:
- Mastering Pivot Table in Python: A Comprehensive Guide
- Data Science Interview Questions Section 3: SQL, Data Warehousing, and General Analytics Concepts
- Data Science Interview Questions Section 2: 25 Questions Designed To Deepen Your UnderstandingÂ
- Data Science Questions Section 1: Data Visualization & BI Tools (Power BI, Tableau, etc.)
- Optum Interview Questions: 30 Multiple Choice Questions (MCQs) with Answers
Hi, I am Vishal Jaiswal, I have about a decade of experience of working in MNCs like Genpact, Savista, Ingenious. Currently i am working in EXL as a senior quality analyst. Using my writing skills i want to share the experience i have gained and help as many as i can.